Functions in Apps Script
In Apps Script, functions are reusable blocks of code that perform specific tasks. Just like building blocks, you can combine functions to create more complex applications. Functions are fundamental to writing organized, maintainable code.
Prerequisites
This tutorial assumes that you have completed the previous tutorials in this series OR are familiar with the following concepts:
What is a Function?
A function is a piece of code that has a name. You can run (or execute) this piece of code elsewhere in your program by using its name. This enables reuse of code. Instead of typing the same code in multiple places, you can define a function in one place and then run it from other places in your program. Functions can:
Accept input values (called parameters)
Process those values using the code inside the function
Return an output value
Be reused throughout your program
Functions in Apps Script have the following structure:
function functionName(parameter1, parameter2) {
// Code that processes the parameters
return outputValue;
}
Let's break down each part:
The keyword
function
tells Apps Script you're creating a functionfunctionName
is what you'll use to run this function elsewhere in your codeParameters in parentheses
()
are values the function needs to do its jobCode between curly braces
{}
is what runs when the function is calledThe
return
statement specifies what value the function gives back
What are Keywords?
In Apps Script, there are a few words that have a special meaning. These words are called keywords. You can see the full list of keywords here.
You don't need to memorize this list. Just remember that Apps Script places some restrictions on these words. For example, you cannot use a keyword as the name of a function.
Keywords are a subset of a larger set of words called reserved words. You also cannot use a reserved word as the name of a function.
Function Parameters
Parameters are like variables that hold the values passed to your function. They:
Are listed in parentheses after the function name
Are separated by commas if there are multiple parameters
Are optional - functions don't need to have parameters
// Function with no parameters
function sayHello() {
return "Hello!";
}
// Function with multiple parameters
function calculateRectangleArea(width, height) {
return width * height;
}
Note
The line in the above code that starts with //
is a comment. Apps Script ignores comments. They are used to help others understand your code.
Return Values
Functions can send back (return) a value that can be used elsewhere in your code. The return
keyword tells Apps Script that you're about to return a value from the function. A function can only return a maximum of one value but you can have functions that don't return any value.
function functionName (inputValue1, inputValue2, inputValue3) {
//The input values are processed to generate the output
return outputValue;
}
Some key points about return values:
Use the
return
keyword to specify what value to send backA function stops executing when it hits a return statement
Functions can return any type of value (number, string, boolean, etc.)
Functions don't have to return anything (these are called void functions)
What is a statement?
A statement is a line of code in Apps Script that does something. Statements usually end with a semicolon. In the previous para, by "return statement", I meant the following line of code:
return outputValue;
Here is a diagram that visualizes what the above function is doing:
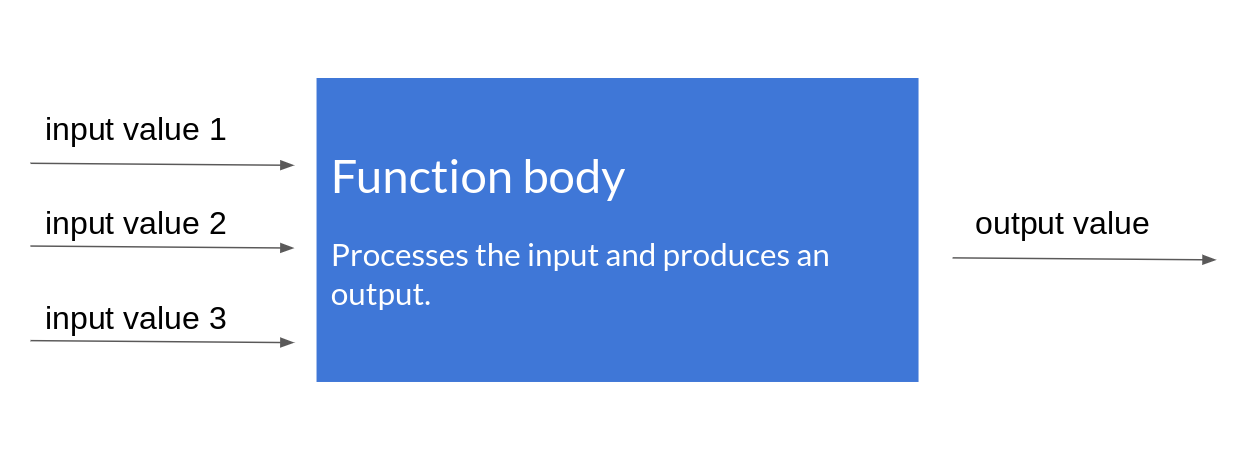
function functionName (inputValue1, inputValue2, inputValue3) {
}
There are functions that don't need input values. Similarly, you can have functions that don't return any value. It all depends on what the function does.
A function that does not accept any input value but returns an outputValue.
function functionName () {
return outputValue;
}
A function that neither accepts input values nor returns any value.
function functionName () {
}
Naming your function
You must follow certain rules while naming your function.
Function names can only contain letters, numbers, the dollar sign ($) and underscores.
Function names cannot be a keyword.
Function names cannot start with a number.
Remember that function names in Apps Script are case-sensitive. So, MYFUNCTION
is not the same as myFunction
.
Here are some best practices for naming your function.
Use a descriptive name to help future readers of your code understand what the function does.
If the name is made up of several words, either use camel case or use underscores to separate the words. If you choose to separate the words using underscores, ensure that the words are in lower case.
Why Use Functions?
Functions help you organize your code and make it more efficient. Here are the key benefits:
Code Reuse: Instead of writing the same code multiple times, you can write it once as a function and reuse it.
Modularity: Each function can focus on one specific task, making your code easier to understand and maintain.
Organization: Functions help break down complex problems into smaller, manageable pieces.
Creating Your First Function
Let's create a simple function that calculates a student's grade percentage based on their score and the total possible points.
function calculatePercentage(score, totalPoints) {
let percentage = (score / totalPoints) * 100;
return percentage;
}
To use this function:
let studentScore = calculatePercentage(85, 100);
Logger.log(studentScore); // Outputs: 85.0
Best Practices for Writing FunctionsUse descriptive function names that indicate what the function does
Keep functions focused on a single task
Keep functions relatively short and manageable
Use clear parameter names
Add comments to explain complex logic
Return consistent data types
Why Should Your Functions Return Consistent Data Types?
When writing functions, it's important to ensure they return the same type of data every time they run. This makes your functions more reliable and easier to use. Here's what this means:
// ❌ Bad: Returns different types
function getStudentStatus(score) {
if (score >= 70) {
return true; // Returns a boolean
} else {
return "Failed"; // Returns a string
}
}
// ✅ Good: Always returns the same type (string)
function getStudentStatus(score) {
if (score >= 70) {
return "Passed"; // Returns a string
} else {
return "Failed"; // Returns a string
}
}
Why is this important?
Makes your code more predictable
Prevents errors when using the function's return value
Makes it easier for others to use your functions
Helps prevent bugs in your applications
Common Mistakes to AvoidUsing a function before defining it
Forgetting to return a value when one is needed
Trying to use parameters outside the function
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
Exclusive Google Sheets automation tutorials and hands-on exercises
Ready-to-use scripts and templates that transform hours of manual work into seconds
Email updates with new automation tips and time-saving workflows
By subscribing, you agree to our Privacy Policy and Terms of Service
Use descriptive function names that indicate what the function does
Keep functions focused on a single task
Keep functions relatively short and manageable
Use clear parameter names
Add comments to explain complex logic
Return consistent data types
Why Should Your Functions Return Consistent Data Types?
When writing functions, it's important to ensure they return the same type of data every time they run. This makes your functions more reliable and easier to use. Here's what this means:
// ❌ Bad: Returns different types
function getStudentStatus(score) {
if (score >= 70) {
return true; // Returns a boolean
} else {
return "Failed"; // Returns a string
}
}
// ✅ Good: Always returns the same type (string)
function getStudentStatus(score) {
if (score >= 70) {
return "Passed"; // Returns a string
} else {
return "Failed"; // Returns a string
}
}
Why is this important?
Makes your code more predictable
Prevents errors when using the function's return value
Makes it easier for others to use your functions
Helps prevent bugs in your applications
Using a function before defining it
Forgetting to return a value when one is needed
Trying to use parameters outside the function
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
By subscribing, you agree to our Privacy Policy and Terms of Service