Loops in Apps Script
Imagine that you have an array containing the names of all students in a class.
let names = [
"Kirstin Gallant",
"Joe Sarf",
"Mark Walling",
"Jane Li",
"Xu Jiang",
"Ramesh Krishna",
"Wei Tu",
"Sandra Westgate",
"Gerardo Riviera"
];
Since there are nine students, we will need to use nine statements to log all of their names.
Logger.log(names[0]);
Logger.log(names[1]);
Logger.log(names[2]);
Logger.log(names[3]);
Logger.log(names[4]);
Logger.log(names[5]);
Logger.log(names[6]);
Logger.log(names[7]);
Logger.log(names[8]);
The more students there are, the more statements we'll need. Imagine trying to log the names of every student in a school. There could be hundreds of students. This can become cumbersome so there has got to be a better way to do this.
Introducing loops in Apps Script
A loop is a piece of code that runs a set of statements multiple times. Each run is called an iteration. There are two common types of loops in Apps Script.
For loop: it is used to run a set of statements a certain number of times. A For loop is usually used when you know the number of iterations in advance.
While loop: it is used to keep running a set of statements as long as some condition is met. A While loop is used in situations where you don't know the number of iterations in advance.
The For loop
A For loop is usually used to implement some type of counter in your program. In the above example, we used nine statements to print the names of students in a class.
The only change in every statement was the array index (0, 1, 2 … 8). Instead of using nine separate statements, you could have used just this one statement:
Logger.log(names[counter]);
Here, counter
is a variable whose value should start at 0 (first student) and should increase by 1 in each iteration until it reaches 8 (last student).
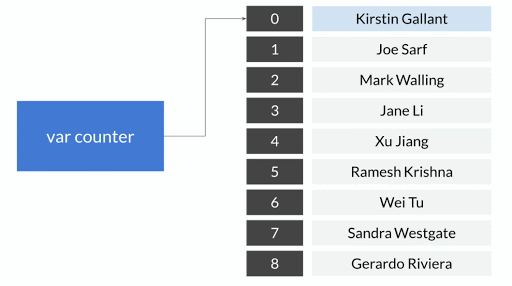
Here is how you'd implement this using a For loop.
for (let counter = 0; counter <= 8; counter = counter + 1) {
Logger.log(names[counter]);
}
Let's unpack how it works. A For loop has four steps.
Initialize the counter variable.
Check if the specified condition is met.
If the condition is met, run the statements inside the loop (within the curly brackets).
Increment the counter and proceed to the next iteration.
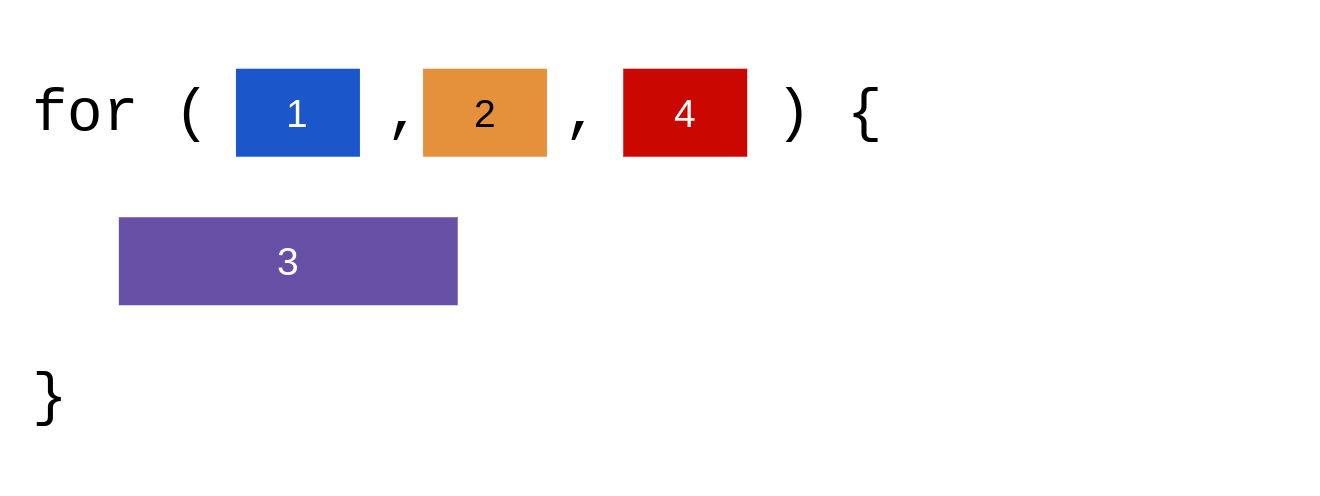
Here is a flowchart diagram of the four steps in a For loop.
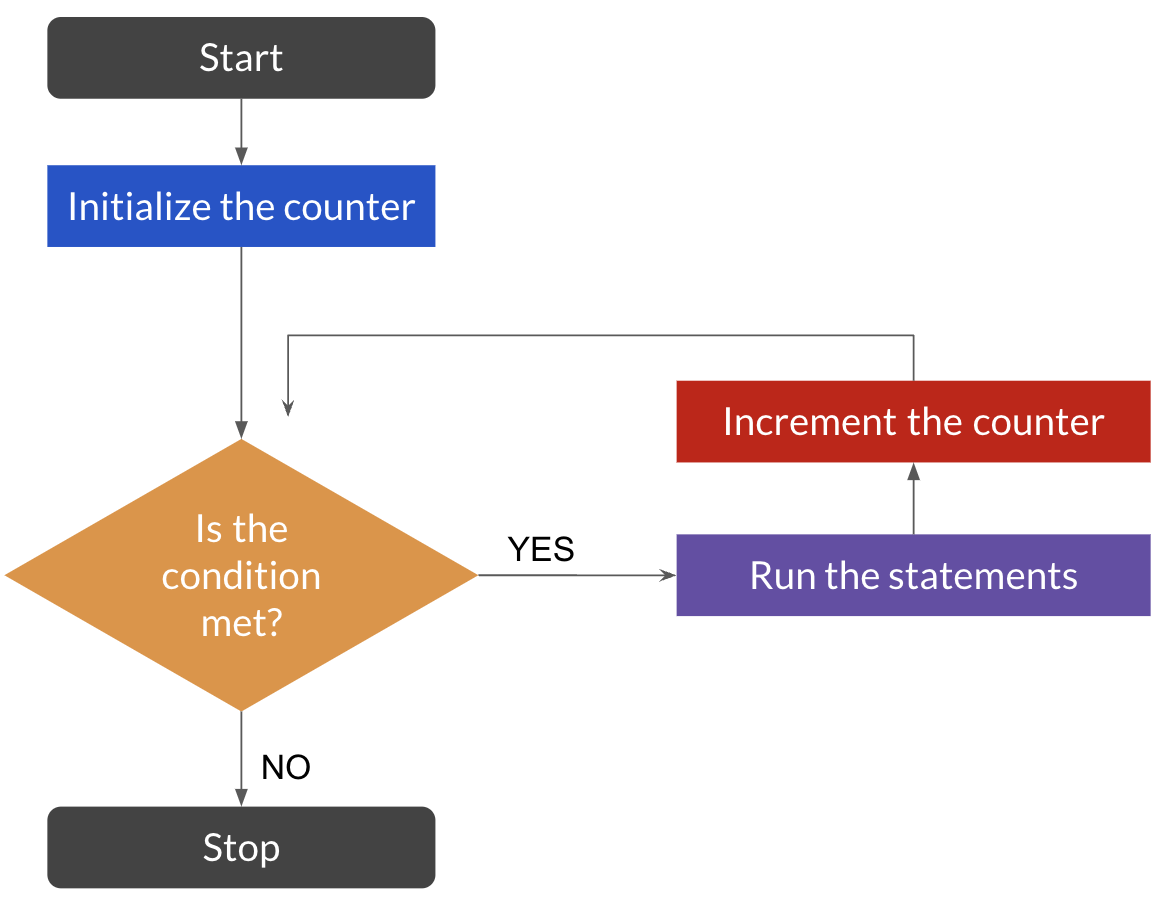
A step-by-step For loop example
The table below shows you how the following For loop works.
for (let counter = 0; counter <= 8; counter = counter + 1) {
Logger.log(names[counter]);
}
Iteration | Counter value | Is condition met? | Statements that are run |
---|---|---|---|
1 | 0 (counter initialized to 0) | 0 <= 8 is TRUE | Logger.log(names[0]); |
2 | 1 | 1 <= 8 is TRUE | Logger.log(names[1]); |
3 | 2 | 2 <= 8 is TRUE | Logger.log(names[2]); |
4 | 3 | 3 <= 8 is TRUE | Logger.log(names[3]); |
5 | 4 | 4 <= 8 is TRUE | Logger.log(names[4]); |
6 | 5 | 5 <= 8 is TRUE | Logger.log(names[5]); |
7 | 6 | 6 <= 8 is TRUE | Logger.log(names[6]); |
8 | 7 | 7 <= 8 is TRUE | Logger.log(names[7]); |
9 | 8 | 8 <= 8 is TRUE | Logger.log(names[8]); |
10 | 9 | 9 <= 8 is FALSE (STOP) |
The While loop
Imagine that you're writing a program to guess a secret number. Let's say that the secret number is 55. In every iteration, your program generates a number between 0 and 100. If this number is equal to 55, the program ends. Otherwise, it proceeds to the next iteration and guesses the next number.
It is hard to use a For loop here because you don't know how many guesses will be needed before your program correctly guesses 55. This is where a While loop becomes handy.
The code below shows you how to use a While loop. The code keeps guessing a number between 0 and 100 until it correctly guesses 55. It also uses the numGuesses
variable to track how many guesses it took to guess the secret number correctly.
The expression Math.floor(100 * Math.random())
generates a random number between 0 and 100. The function Math.random()
generates a random number between 0 and 1. Let's say it generates 0.16251661. Multiplying it by 100 will result in a number between 0 and 100. The number 0.16251661 becomes 16.251661 when it is multiplied by 100. Finally, the function Math.floor
rounds the number down to the nearest integer. So Math.floor(16.251661)
results in 16.0.
function guess() {
let numGuesses = 1;
let guess = Math.floor(100 * Math.random());
while (guess != 55) {
numGuesses = numGuesses + 1;
guess = Math.floor(100 * Math.random());
}
Logger.log(numGuesses);
}
The structure of a While loop is simpler than a For loop. There are just two steps.
Check if a condition is met.
If it is met, run the statements inside the While loop. Then repeat Step 1.
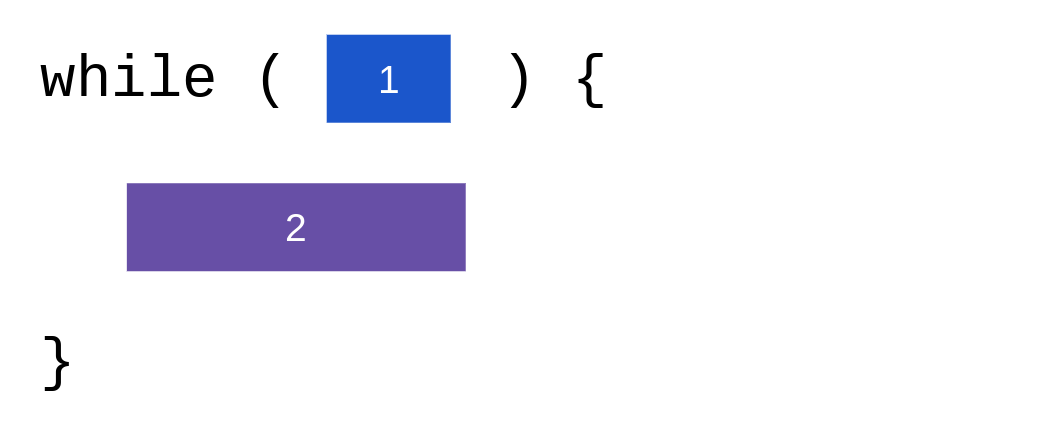
Here is a flow chart diagram of the steps in a While loop.
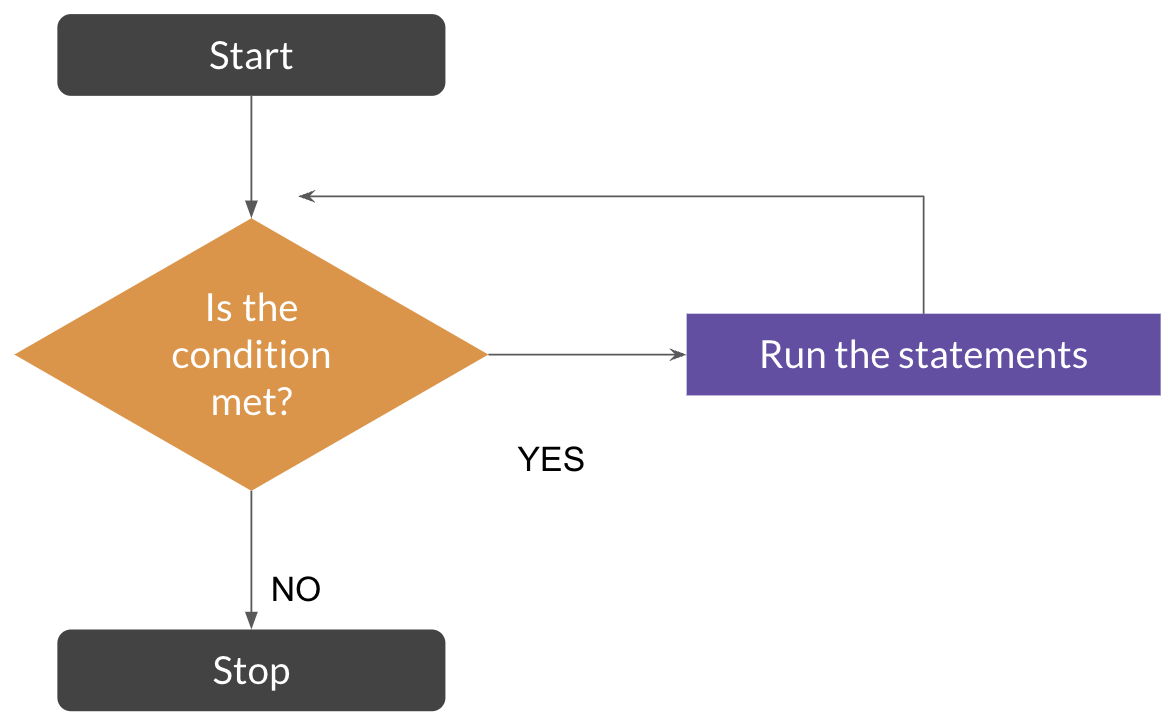
A step-by-step While loop example
The table below shows you how the following For loop works.
let numGuesses = 1;
let guess = Math.floor(100 * Math.random());
while (guess != 55) {
numGuesses = numGuesses + 1;
guess = Math.floor(100 * Math.random());
}
Iteration | Value of numGuesses and guess | Is the condition met? | Statements that are run |
---|---|---|---|
1 | numGuesses = 1 guess = 16.0 | 16.0 != 55 is TRUE | numGuesses = numGuesses + 1; (numGuesses becomes 2) guess = Math.floor(100 * Math.random()); (Assume that guess becomes 23.0) |
2 | numGuesses = 2 guess = 23.0 | 23.0 != 55 is TRUE | numGuesses = numGuesses + 1; (numGuesses becomes 3) guess = Math.floor(100 * Math.random()); (Assume that guess becomes 71.0) |
3 | numGuesses = 3 guess = 71.0 | 71.0 != 55 is TRUE | numGuesses = numGuesses + 1; (numGuesses becomes 4) guess = Math.floor(100 * Math.random()); (Assume that guess becomes 9.0) |
4 | numGuesses = 4 guess = 9.0 | 9.0 != 55 is TRUE | numGuesses = numGuesses + 1; (numGuesses becomes 5) guess = Math.floor(100 * Math.random()); (Assume that guess becomes 55.0) |
5 | numGuesses = 5 guess = 55.0 | 55.0 != 55 is FALSE STOP |
In the above example, it took 5 guesses for the program to correctly guess 55. If you run the program again, the number of guesses will likely be different. It is impossible to know the number of guesses in advance. What we do know in advance is when to stop. We know we should stop when we correctly guess the secret number. This is the difference between a For and While loop. Use a For loop if you know the number of iterations in advance. Use a While loop if you know when to stop based on some condition but you have no idea how many iterations it will take for this condition to be met.
The Do...While Loop
The do...while
loop is a variant of the while loop with one key difference - the condition is checked at the end of the loop rather than at the beginning. This means the code block will always execute at least once before the condition is evaluated.
Here's the basic structure:
do {
// Code block to be executed
} while (condition);
Let's modify our number guessing example to use a do...while loop:
function guess() {
let numGuesses = 1;
let guess;
do {
guess = Math.floor(100 * Math.random());
Logger.log("Guessing: " + guess);
} while (guess != 55);
Logger.log("Found it in " + numGuesses + " guesses!");
}
When to Use Do...While vs While
The choice between do...while and while loops depends on whether you want to guarantee at least one execution of the loop body:
Use
while
when you need to check the condition before the first executionUse
do...while
when you want to ensure the loop body runs at least once
Here's a practical example showing the difference:
// Using while loop
let count = 10;
while (count < 10) {
Logger.log(count); // Never executes
count++;
}
// Using do...while loop
let count = 10;
do {
Logger.log(count); // Executes once
count++;
} while (count < 10);
Common Use Cases for Do...While
Do...while
loops are particularly useful in scenarios where you need to:
Process user input at least once
Validate data with a minimum first attempt
Execute a task that must happen at least one time
Best PracticesAlways include a way to eventually break out of the loop to avoid infinite execution
Consider readability - sometimes a while loop with initial setup can be clearer
Use meaningful variable names that make the loop's purpose obvious
Add comments to explain complex loop conditions
Always include a way to eventually break out of the loop to avoid infinite execution
Consider readability - sometimes a while loop with initial setup can be clearer
Use meaningful variable names that make the loop's purpose obvious
Add comments to explain complex loop conditions
Now that we've covered all three types of loops (for, while, and do...while), you can choose the most appropriate one for your specific use case. Let's move on to learning about break and continue statements.
Break and continue statements
The break and continue statements can be used to jump out of a loop or jump to the next iteration. The code within the loop after one of these statements will NOT be run.
The break statement is used to jump out of the loop. The continue statement is used to jump to the next iteration.
The diagram below illustrates how these statements work with a While loop but they will also work with a For loop in the same way.
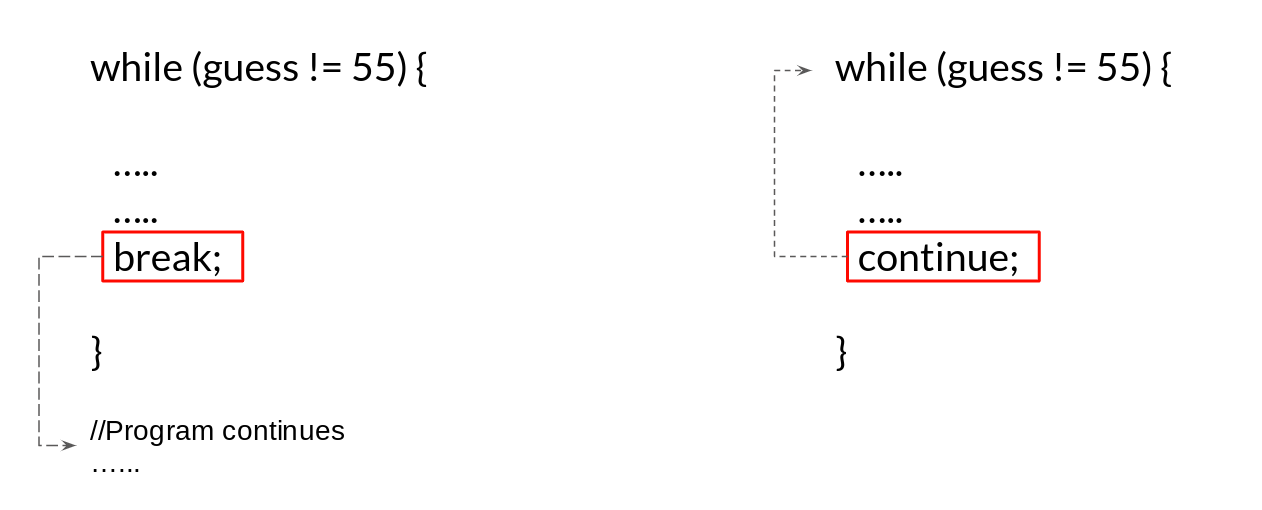
Here is an example of how to use the break statement. Let's say that we only want to try guessing the correct number a certain number of times. The code below uses the break statement to jump out of the loop after guessing the number incorrectly a 1000 times.
In the previous version of the code, the While loop would have ended ONLY upon guessing 55 correctly. In this new version, the While loop can end under two circumstances. Either the correct number is guessed OR it runs out of guesses. Therefore, we need to use an IF statement after the loop to log the correct message. If the While loop ends without guessing 55, it means it ran out of guesses. Otherwise, it guessed the number correctly.
function guess() {
let numGuesses = 1;
let guess = Math.floor(100 * Math.random());
while (guess != 55) {
if(numGuesses === 1000) {
break; //We only want to guess 1000 times
}
numGuesses = numGuesses + 1;
guess = Math.floor(100 * Math.random());
}
if(guess != 55) {
//We ran out of guesses
Logger.log("Could not guess the secret number in 1000 guesses");
} else {
Logger.log(numGuesses);
}
}
How was this tutorial?
Your feedback helps me create better content
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
By subscribing, you agree to our Privacy Policy and Terms of Service