Objects in Apps Script
In Apps Script, objects are used to organize the values in your program that are related to one another.
For example, imagine that you are building an app that is used by teachers in a school to mark attendance. The app needs a way to store and process certain information about each student. Let's say this app needs to display an alphabetically sorted list of students that is organized by grade and section.
In order to do this, the app needs to know the following information about each student.
Their name.
Their grade.
Their section.
You could use several variables to store the above information.
let firstName = "Megan";
let lastName = "Shelton";
let grade = "preschool";
let section = "A";
The above variables are related to each other. They are a specific student's information. So, instead of creating these four variables, you could create an object that contains all of this information. This is what that might look like:
let student = {
firstName : "Megan",
lastName : "Shelton",
section : "A",
grade : "preschool"
}
Just by reading the above code, you can infer that the four pieces of data are related. They're a specific student's information.
An object is a value that contains other values
The values contained within an object are called properties. To make things more complex, every property must have a value associated with it. I will use a diagram to explain this to you.
We'll start with value 1 which is an object. Value 1 contains three values (value 2, value 3 and value 4) and these are called properties of value 1. Each property has an associated value. So, for example, value 2 has value 5 associated with it and value 3 has value 6 associated with it.
In the diagram, all the boxes are values. The blue boxes are values that are called properties. Each property has a value associated with it.
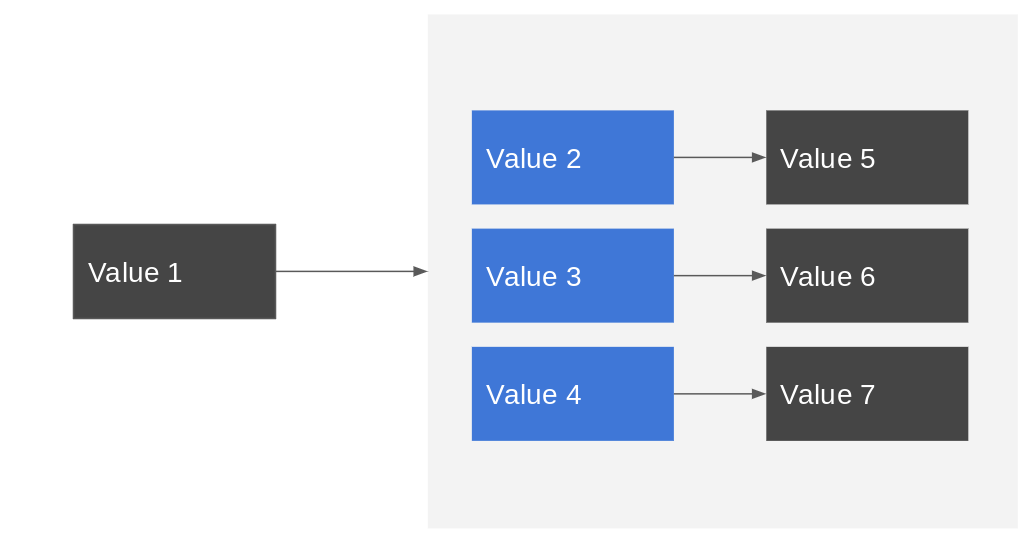
Another way to think about an object is that it contains pairs of values. Each pair consists of a property and its value. In programming, these types of linked values are also called key-value pairs.
Creating an object in Apps Script
You can create an object in Apps Script like this:
let student = {};
Logger.log(student); //{}
The curly brackets tell Apps Script that the variable student contains a value of type object.
Set the properties of the object and their corresponding values by using the dot notation.
let student = {};
student.firstName = "Jane";
student.lastName = "Lee";
student.age = 4;
Logger.log(student); //{firstName=Jane, lastName=Lee, age=4.0}
Once set, you can also access these values using the dot notation.
Logger.log(student.firstName); //Jane
Logger.log(student.lastName); //Lee
Logger.log(student.age); //4.0
Delete properties and their associated values by using the delete operator.
let student = {};
student.firstName = "Jane";
student.lastName = "Lee";
student.age = 4;
Logger.log(student.age); //4.0
delete student.age;
Logger.log(student.age); //undefined
Set multiple properties at once in the following way:
let student = {
firstName : "Megan",
lastName : "Shelton",
section : "A",
grade : "preschool"
}
Note
When you set multiple properties at once, remember to use a colon (:) to separate the property and its value. Also, remember to use a comma after every property-value pair. There is, however, no comma needed after the last property-value pair. Notice that I did not add a comma after grade: "preschool"
.
Brackets notation
You can access a property's value using the brackets notation. The following two statements are equivalent:
student.firstName
//dot notationstudent["firstName"]
//brackets notation - note the quotes!
The brackets notation is particularly useful when the property name is stored in a variable or when the property name contains special characters:
let propertyName = "firstName";
student[propertyName] // This works because propertyName contains "firstName"
// Bracket notation also works with property names that have special characters:
student["first-name"] // Dot notation wouldn't work here: student.first-name
Methods
If the value associated with a property is a function, the property is called a method.
let student = {};
student.firstName = "Jane";
student.lastName = "Lee";
student.age = 4;
student.getParentInfo = function() {
//some code that returns info about the student's parents.
};
Logger.log(student);
In the above code, getParentInfo
is a method of the student object. You can access the method's value (ie, the code for the function) using the dot notation.
student.getParentInfo; //returns the code for the function
Since getParentInfo
is a method, you can also execute its function by using parentheses.
student.getParentInfo; //returns the code for the function
student.getParentInfo(); //executes the function and returns its output value
Checking if an object has a property
Use the in
operator and the name of the property to check if an object contains that property. The name of the property must be enclosed by either single or double quotes.
let student = {
firstName : "Megan",
lastName : "Shelton",
section : "A",
grade : "preschool"
}
Logger.log("firstName" in student); //true
Logger.log("age" in student); //false since the student object does not have a property called age
student.age = 4;
Logger.log("age" in student); //true since we just set the property age in the above line
How was this tutorial?
Your feedback helps me create better content
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
By subscribing, you agree to our Privacy Policy and Terms of Service