Pop up alert messages in Google Sheets
A pop up alert message is used to display important information that the user must pay attention to. They can also be used to confirm with the user before taking some action.
★ Alerts are not the same as prompts
A prompt is used to get input from the user whereas an alert only displays information to the user. Please see the tutorial on getting user input in Google Sheets for more information about prompts.
To display a pop up alert message, you need to write some code using Google Apps Script. Don't worry, it is very simple and straightforward to do that.
Prerequisites
If you've never worked with Apps Script before, I've written an article that explains how to create and run your first apps script. I've also written a series of articles to teach you how to code using Google Sheets and Apps Script.
Google Sheets Custom UI Demo Template
Explore a variety of custom user interface options in Google Sheets! This template demonstrates custom menus, dialogs, sidebars, alerts, prompts, and more, all powered by Google Apps Script. A perfect way to learn and get inspired for your own projects.
Already a subscriber? Log in
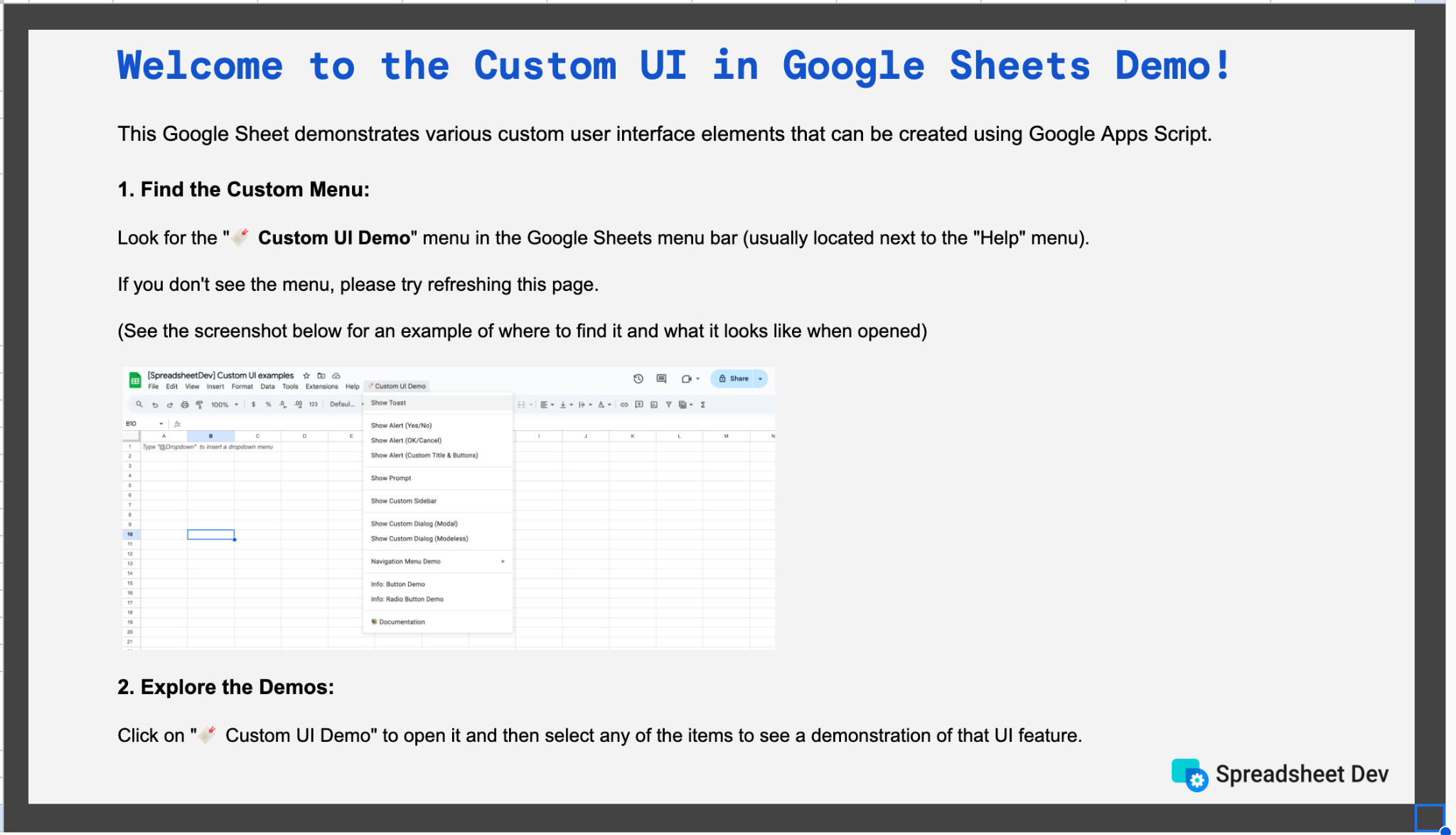
It only takes a few lines of code to display an alert message.
function alertMessage() {
SpreadsheetApp.getUi().alert("Alert message");
}
When you run the above function, you should see the alert message in your spreadsheet.
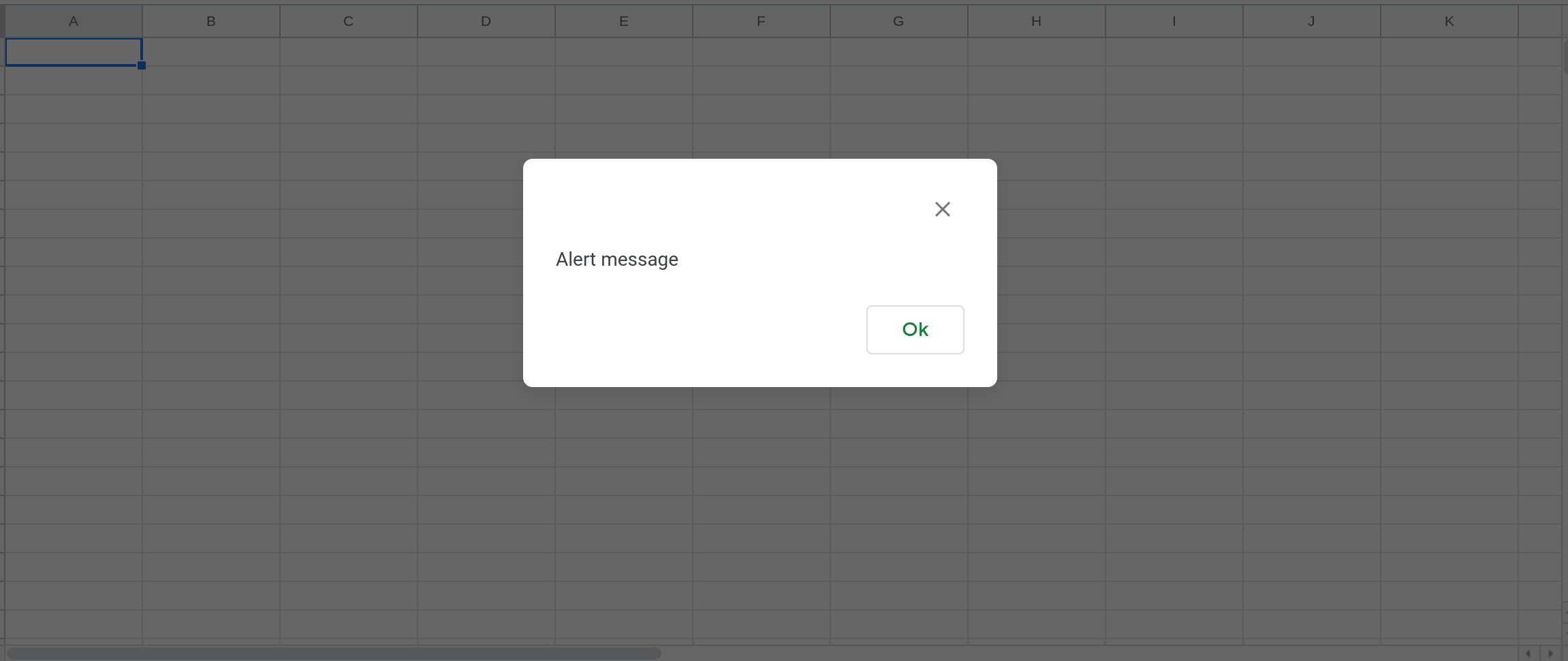
The user can dismiss the alert by selecting the close button (i.e., the [X]) or the [Ok] button. Unlike many other applications, clicking outside the alert will not dismiss it.
The alert()
function will return the button that the user selected. The script can use this information to decide how to proceed. For example, the script might take some action only if the user selected [Ok].
function alertMessage() {
var result = SpreadsheetApp.getUi().alert("Alert message");
if(result === SpreadsheetApp.getUi().Button.OK) {
//Take some action
SpreadsheetApp.getActive().toast("About to take some action …");
}
}
Specify what buttons to display on the alert dialog
Sometimes you might want the user to select YES or NO to a proposed course of action. An alert message can be configured to display a specific set of buttons. The alert()
function will return the button that the user selected so the script can take the appropriate course of action.
The button sets supported by alert dialogs are:
OK button
OK / CANCEL buttons
YES / NO buttons
YES / NO / CANCEL buttons
Displaying an alert message with an OK button
Use this button set for informational alerts. Please note that this is the button set that will be used by default when you do not specify the button set to be used.
🛈 Note
From a user experience point of view, selecting [X] or [Ok] should both result in the same action being taken by the script. If different actions need to be taken based on the user's selection, please use one of the other button sets below.
function alertMessageOKButton() {
var result = SpreadsheetApp.getUi().alert("Alert message", SpreadsheetApp.getUi().ButtonSet.OK);
SpreadsheetApp.getActive().toast(result);
}
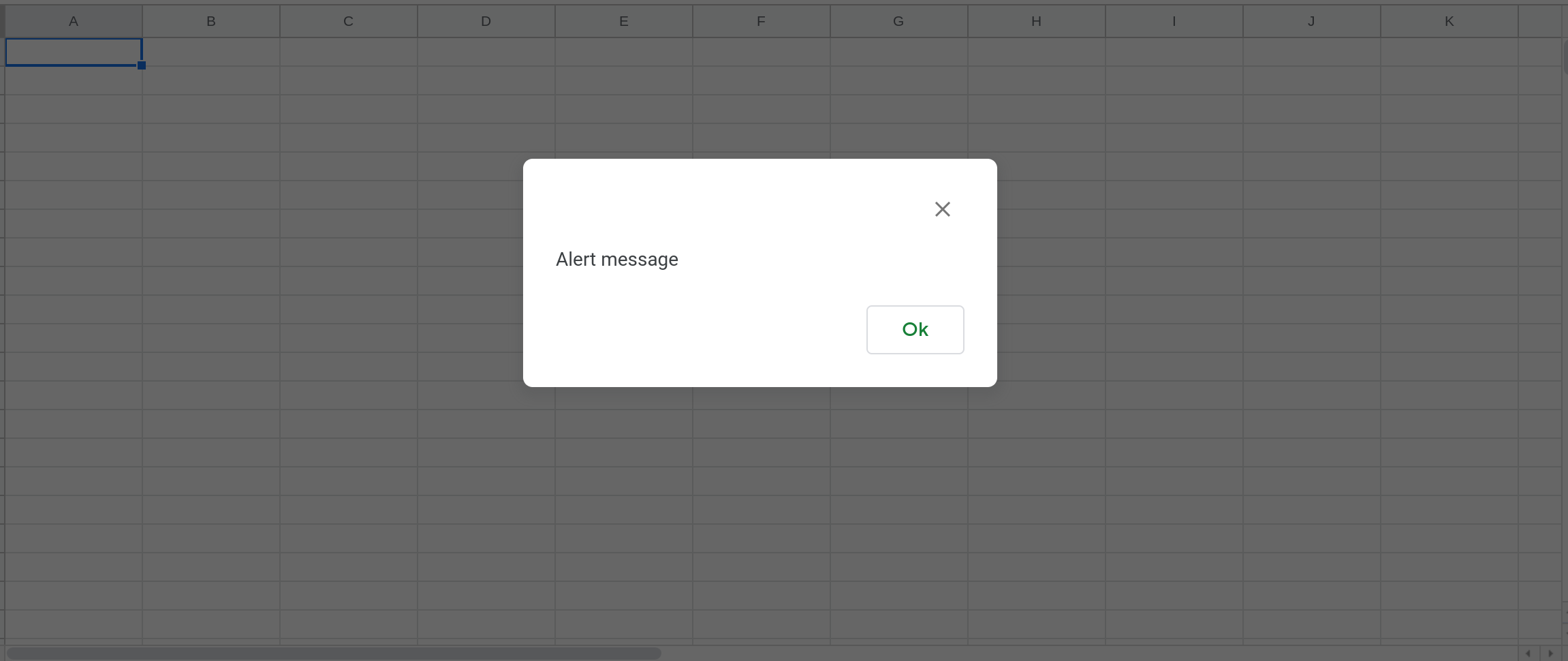
Displaying an alert message with OK/CANCEL buttons
Use this button set to confirm with the user before taking some action. If the user selects [Ok], it means they're OK with proceeding. If they choose [X] or [Cancel], it means they do not want to proceed.
function sendEmail() {
var result = SpreadsheetApp.getUi().alert("You're about to send emails to 53 people.", SpreadsheetApp.getUi().ButtonSet.OK_CANCEL);
SpreadsheetApp.getActive().toast(result);
}
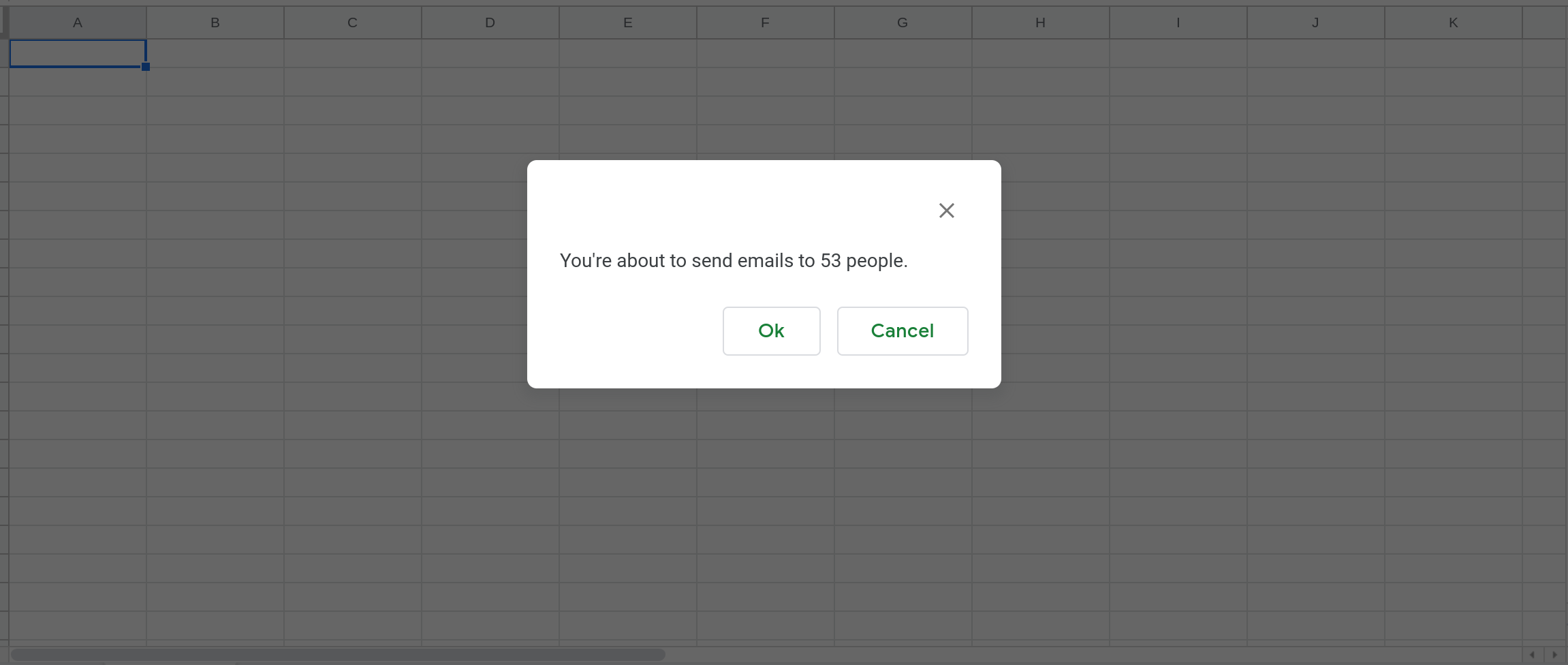
Below is another example of taking a different action based on the button that the user selects.
function sendEmail() {
var result = SpreadsheetApp.getUi().alert("You're about to send emails to 53 people.", SpreadsheetApp.getUi().ButtonSet.OK_CANCEL);
if(result === SpreadsheetApp.getUi().Button.OK) {
//Some code to send out emails
//Let the user know that the emails were sent successfully.
SpreadsheetApp.getActive().toast("53 emails were sent.");
} else {
SpreadsheetApp.getActive().toast("No emails were sent.");
}
}
Displaying an alert message with YES/NO buttons
Use this button set when the user needs to make a Yes or No decision. The user must clearly understand what action will be taken when they select each button.
🛈 Note
If the user selects [X], it does not necessarily mean that they said No. It could also mean that they chose to not make a decision.
function alertMessageYesNoButton() {
var result = SpreadsheetApp.getUi().alert("Jake is requesting 3 days of vacation in June. Do you approve?", SpreadsheetApp.getUi().ButtonSet.YES_NO);
SpreadsheetApp.getActive().toast(result);
}
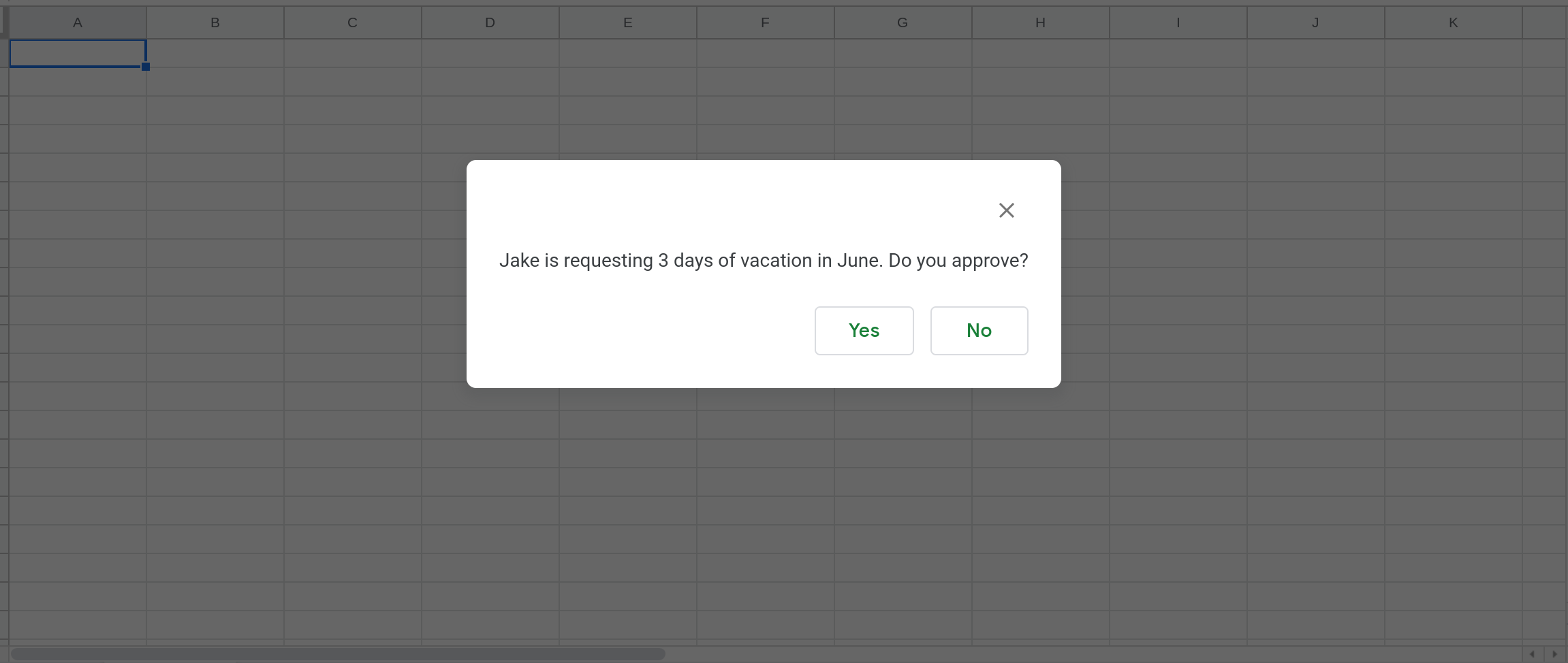
Displaying an alert message with YES/NO/CANCEL buttons
This is similar to the Yes/No button set but makes the [Cancel] option explicit. Again, the user must clearly understand what action will be taken when they select each button. Selecting [X] or [Cancel] must result in the same action being taken.
function alertMessageYesNoCancelButton() {
var result = SpreadsheetApp.getUi().alert("Alert message", SpreadsheetApp.getUi().ButtonSet.YES_NO_CANCEL);
SpreadsheetApp.getActive().toast(result);
}
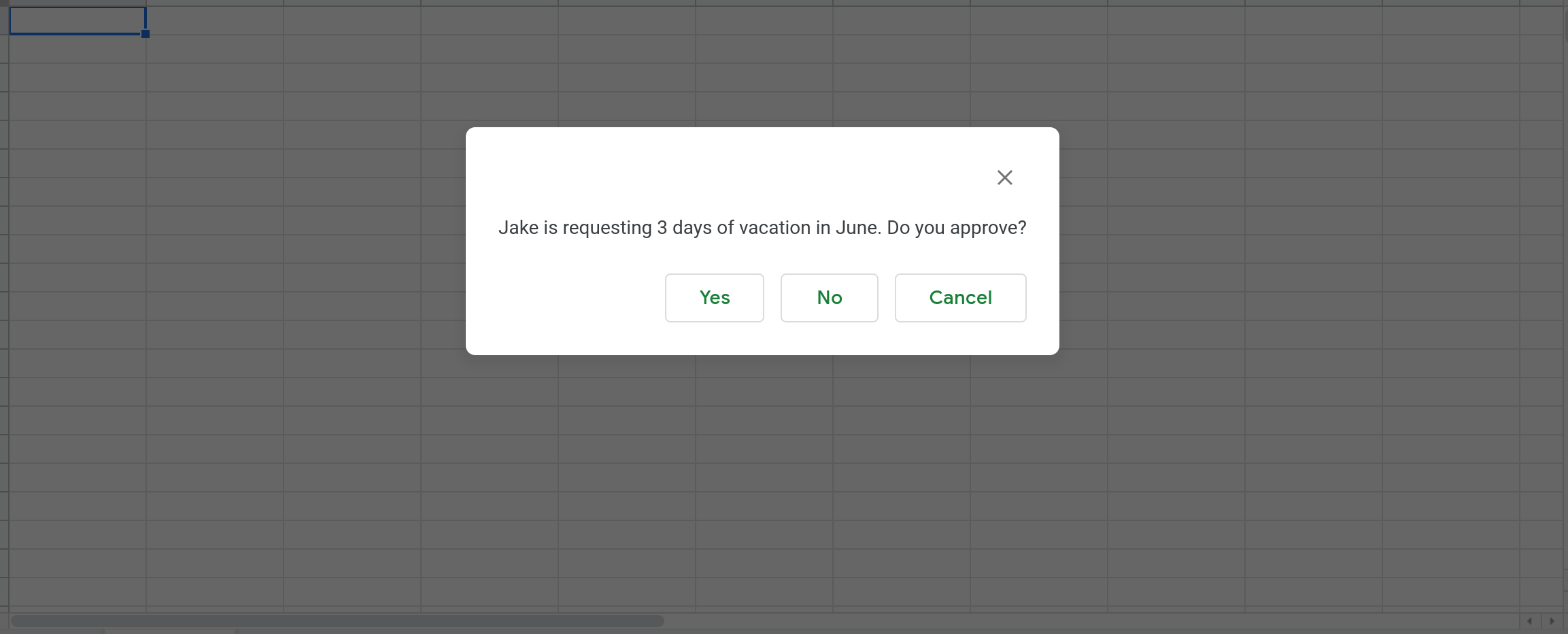
Including a title in the alert dialog
You can specify a title along with the alert message. Please note that you can only specify a title if you also explicitly specify the buttons to be displayed on the alert dialog. Here is an example of how to display an alert message with a title.
function alertMessageTitle() {
SpreadsheetApp.getUi().alert("Title", "Alert message", SpreadsheetApp.getUi().ButtonSet.OK);
}
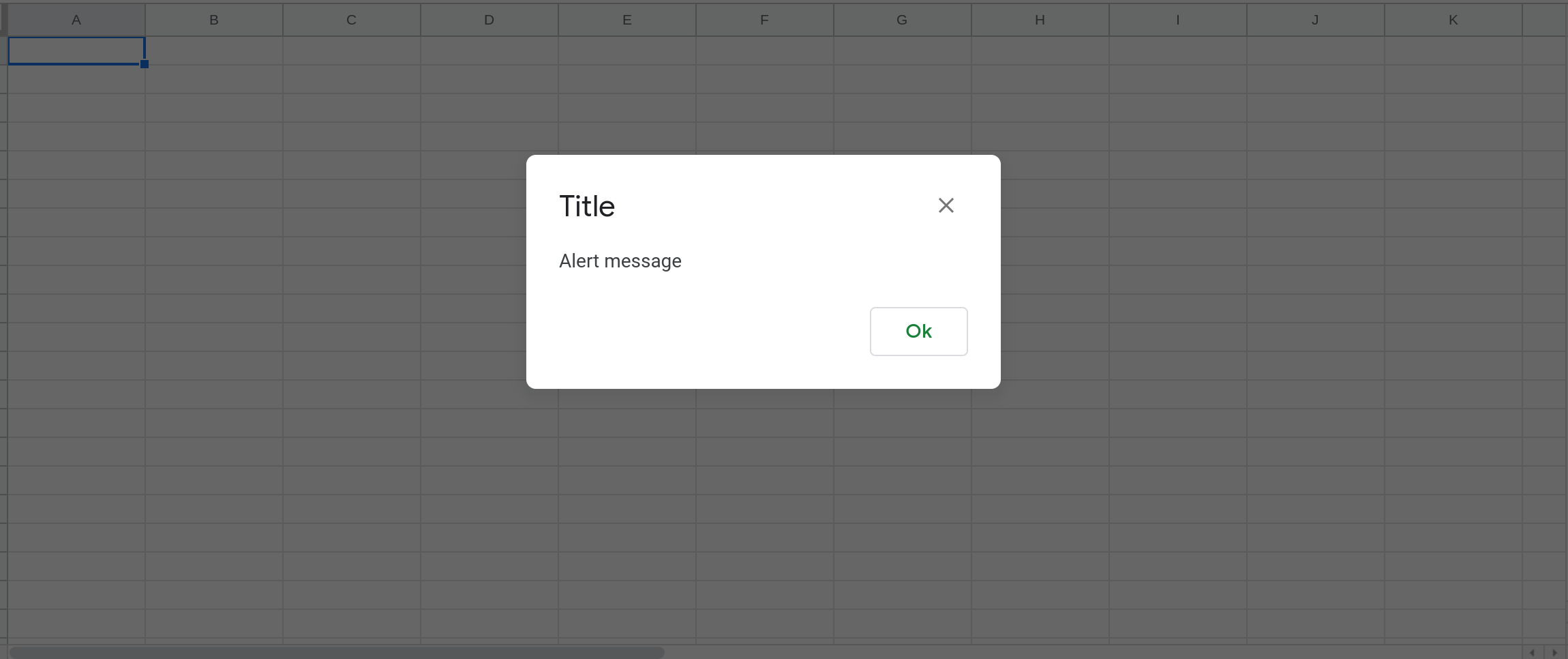
★ Tip: Use emojis (wisely) in alert messages
You can use emojis to make your alerts more meaningful. Here are some emojis that you can consider using:
⚠️: Warning
⏰: Something that is time sensitive
💡: An idea or a recommendation
🎉: An accomplishment
👍: Thumbs up
⚙️: Settings related
The code below shows you how to include emojis in an alert.
function alertMessageEmoji() {
SpreadsheetApp.getUi().alert("⚠️ You're about to share a file externally", "You're about to share this document with bob@example.com, who is not a pre-approved recipient. Are you sure?", SpreadsheetApp.getUi().ButtonSet.YES_NO);
}
Running the above code will display the following alert. The emoji helps the user understand that the alert is warning them about an action that they're about to take.
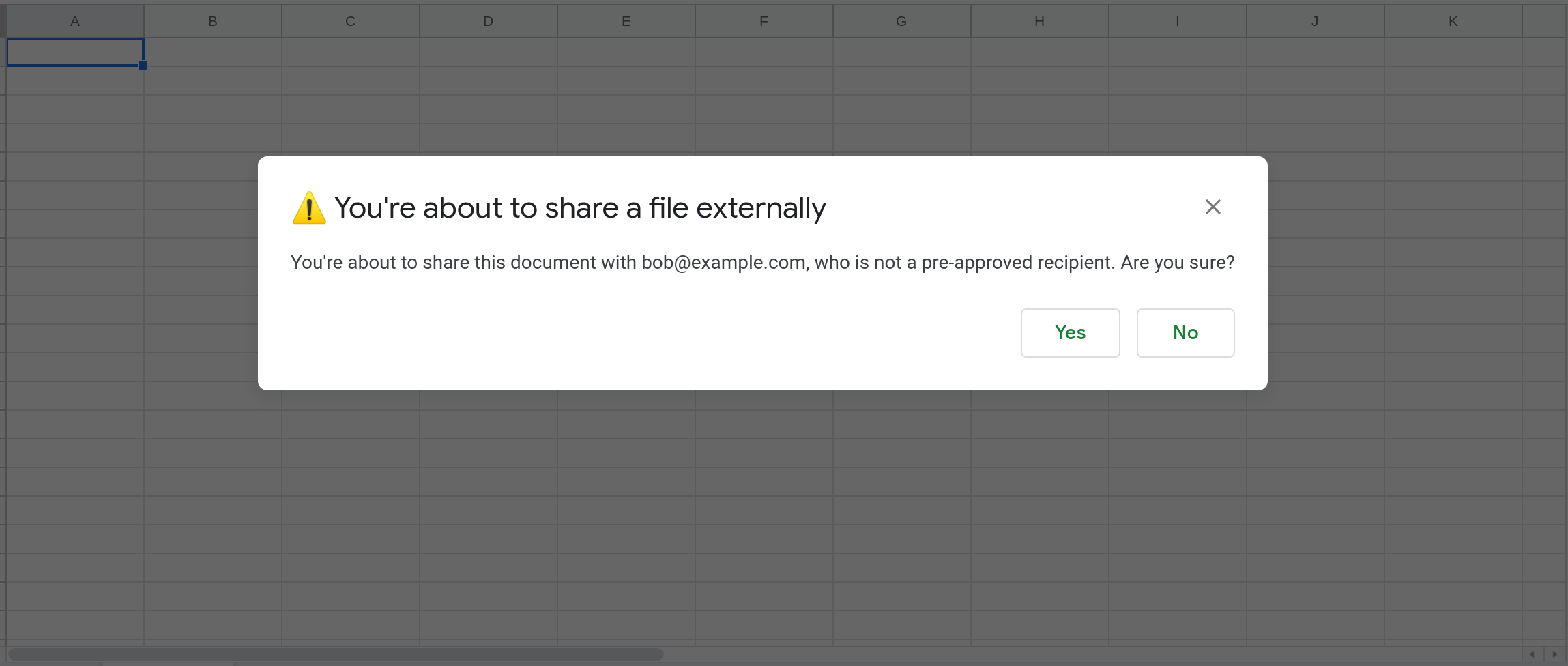
Conclusion
In this tutorial, you learned how to display pop up alert messages in Google Sheets using Google Apps Script.
Thanks for reading.
How was this tutorial?
Your feedback helps me create better content
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
By subscribing, you agree to our Privacy Policy and Terms of Service