Getting user input in Google Sheets using prompts
A prompt is used to get input from the user in a Google Sheets application. A prompt is a popup dialog that has a text field for the user to enter some text.
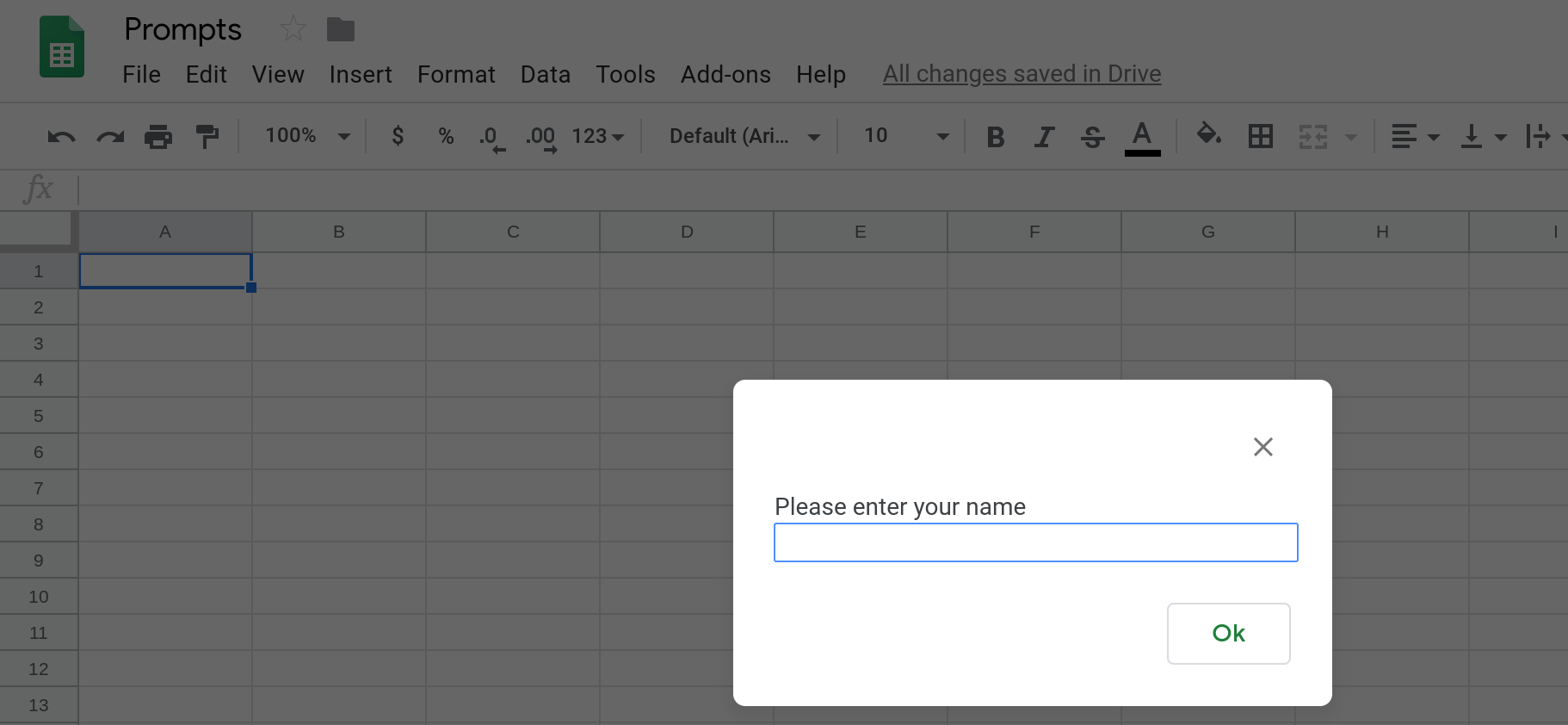
The code to display a prompt is written using Apps Script and is simple to write. For example, the code to display the prompt in the above image is just two lines long.
Note
If you've never worked with Apps Script before, I've written an article that explains how to create your first apps script. I've also written a series of articles to teach you how to code using Google Sheets and Apps Script.
Google Sheets Custom UI Demo Template
Explore a variety of custom user interface options in Google Sheets! This template demonstrates custom menus, dialogs, sidebars, alerts, prompts, and more, all powered by Google Apps Script. A perfect way to learn and get inspired for your own projects.
Already a subscriber? Log in
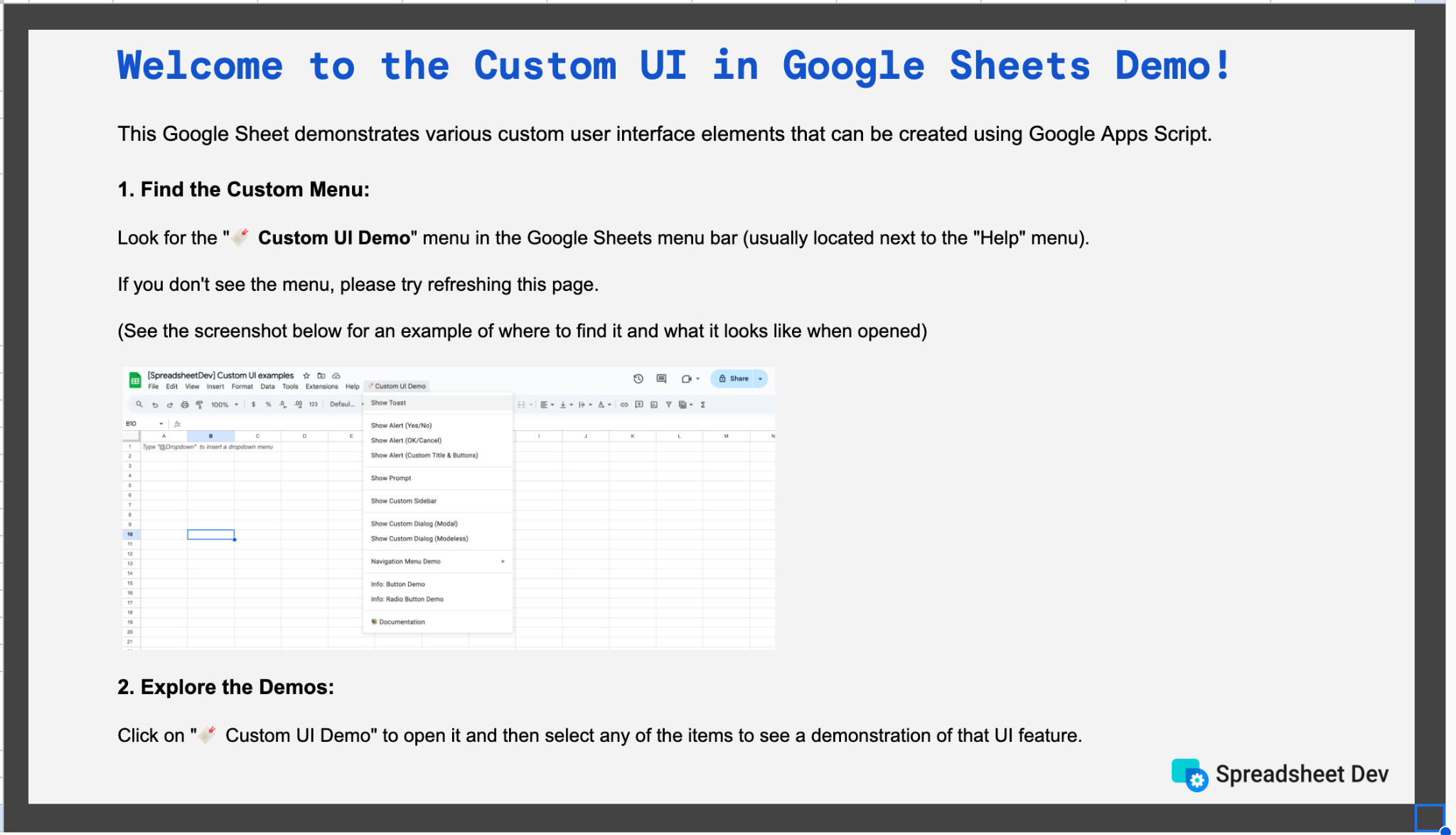
function displayPrompt() {
var ui = SpreadsheetApp.getUi();
var result = ui.prompt("Please enter your name");
}
When the user clicks [OK], you can get the text they entered from your script using the getResponseText()
method.
function displayPrompt() {
var ui = SpreadsheetApp.getUi();
var result = ui.prompt("Please enter your name");
Logger.log(result.getResponseText());
}
You can also find out if the user closed the prompt dialog (ie, they clicked the [X]) using the getSelectedButton()
method.
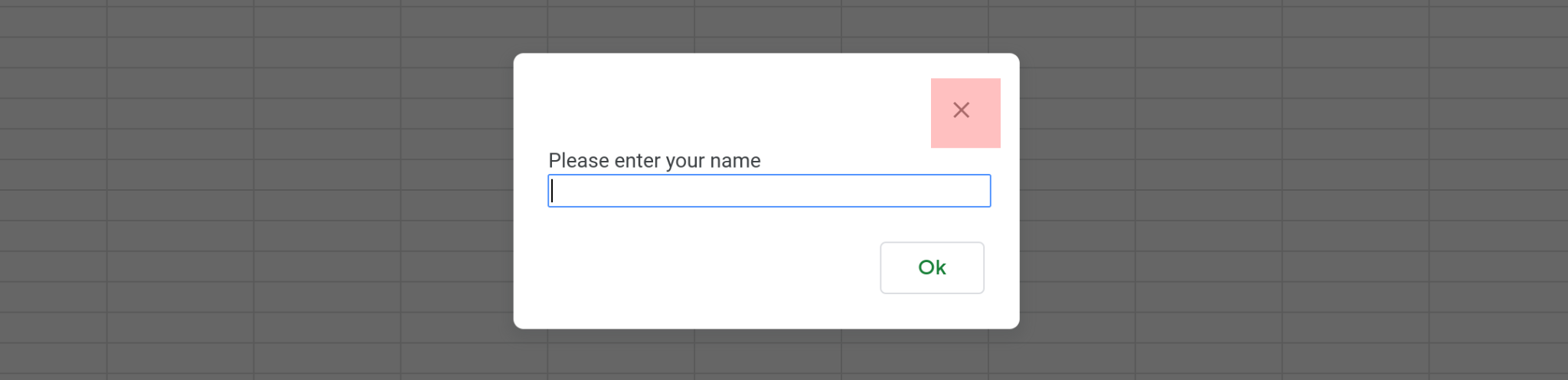
function displayPrompt() {
var ui = SpreadsheetApp.getUi();
var result = ui.prompt("Please enter your name");
//Get the button that the user pressed.
var button = result.getSelectedButton();
if (button === ui.Button.OK) {
Logger.log("The user clicked the [OK] button.");
Logger.log(result.getResponseText());
} else if (button === ui.Button.CLOSE) {
Logger.log("The user clicked the [X] button and closed the prompt dialog.");
}
}
To display the prompt, click the play icon in the script editor.
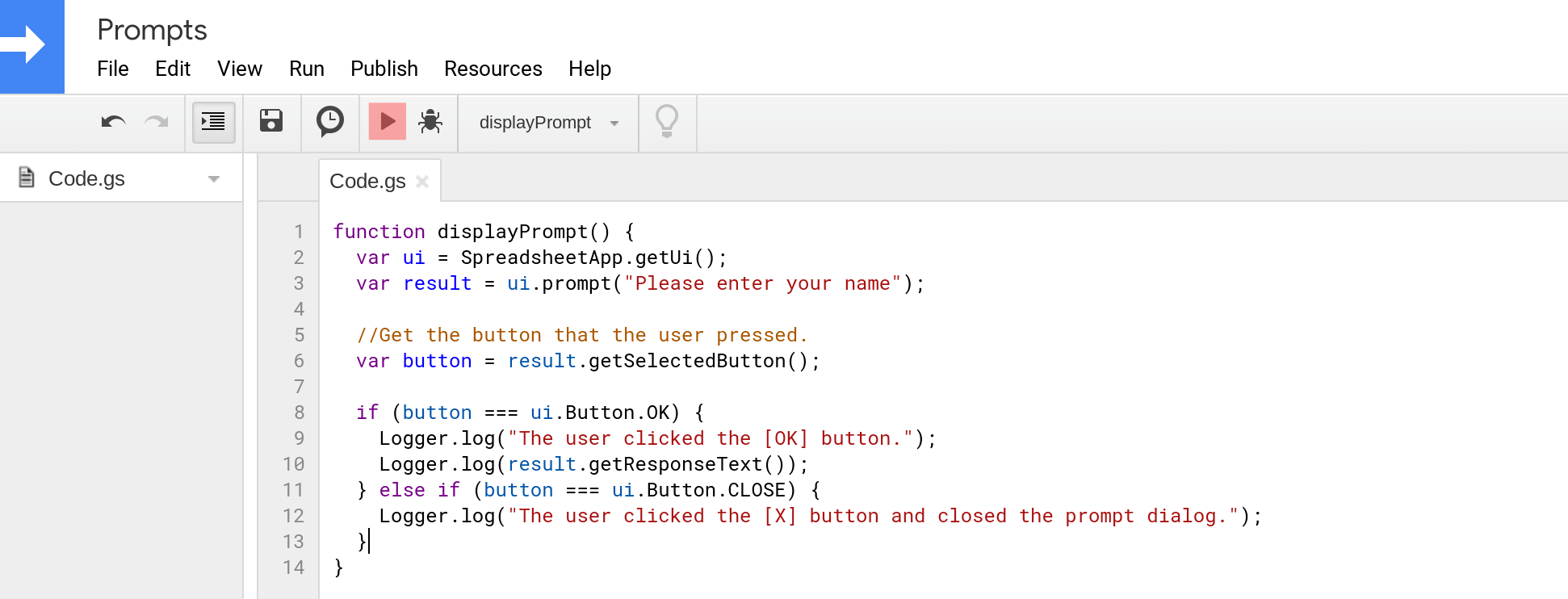
When you click play, the prompt will be displayed in the Google sheet that the script belongs to.
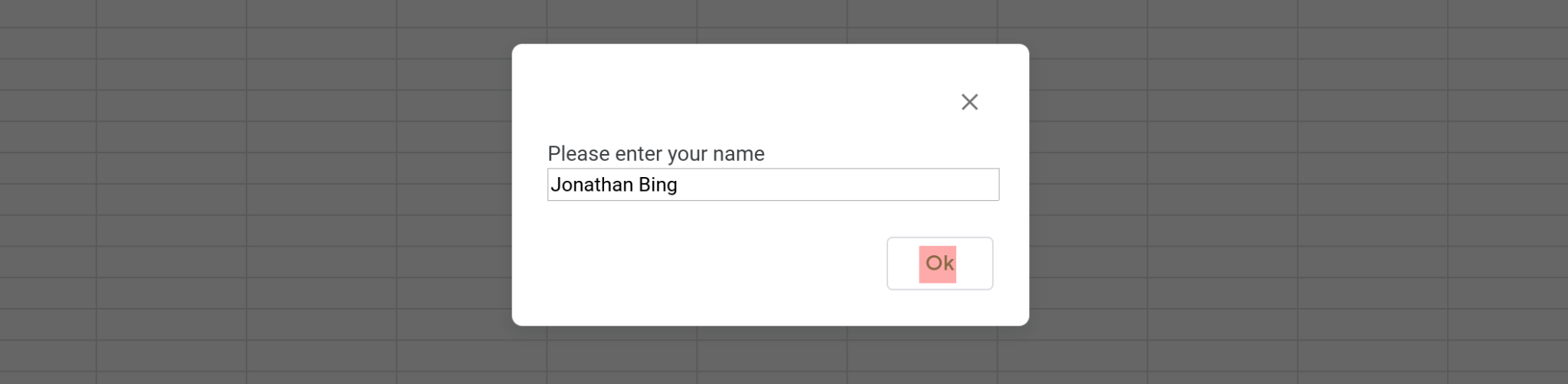
You'll see the name you enter in the script's logs. To view these logs, click View →Logs.
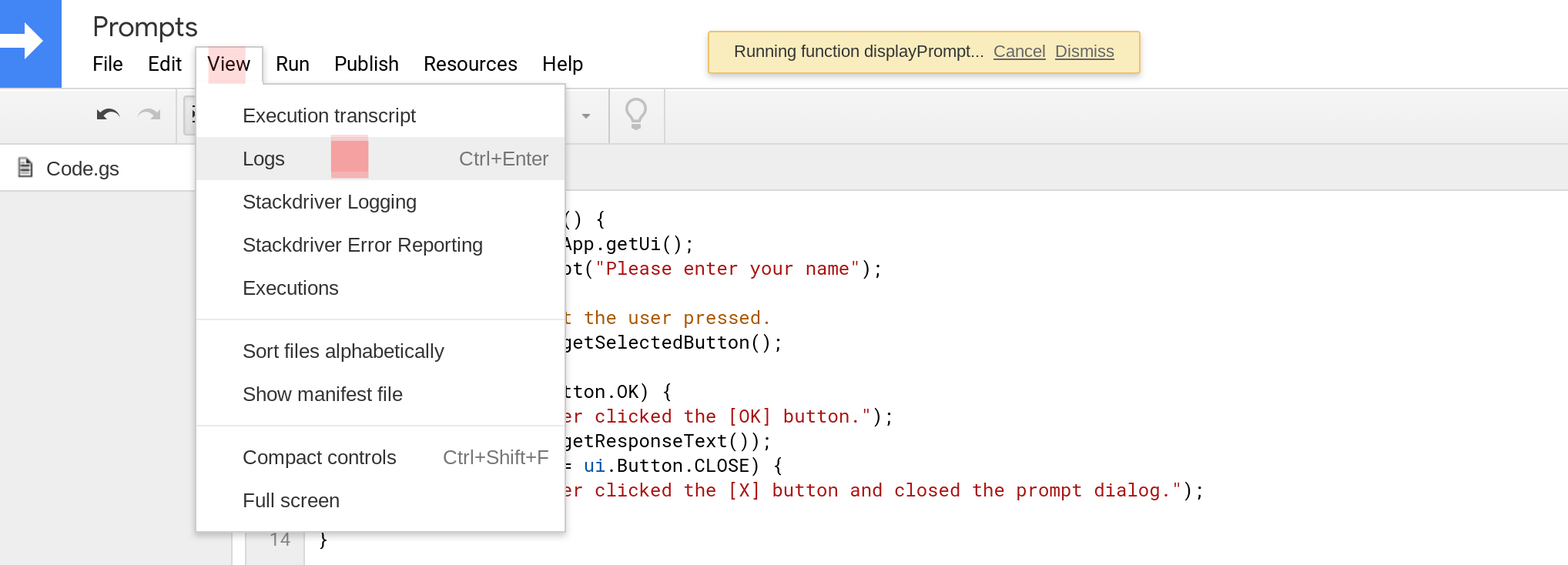
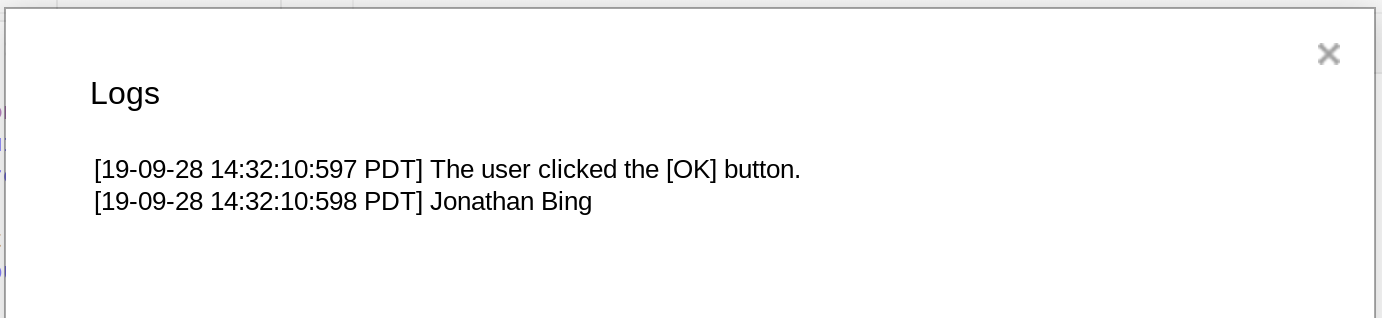
Once you get input from the user, what you do with it will depend on the application you are writing.
Summary
In this article, you learned how to get input from the user using a prompt dialog.
Thanks for reading.
How was this tutorial?
Your feedback helps me create better content
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
By subscribing, you agree to our Privacy Policy and Terms of Service