Create a Custom Function to Identify Email Providers in Google Sheets
Have you ever needed to categorize email addresses by their provider? Whether you're analyzing your marketing campaign data or organizing your contact list, knowing the email provider can be invaluable. In this tutorial, I'll show you how to create a custom function in Google Sheets that automatically detects the email provider by analyzing the domain's MX records. We will use Google's public DNS API to look up the MX (mail exchanger) records for the domain (not sure what an API is? Check out my beginner-friendly explanation). These records tell us which mail servers handle email for the domain.
What are MX records?
Before beginning this tutorial, it's helpful to know a little about MX records. Think of them like the postal service for email. Every email address has a 'domain' – the part after the @ symbol (like gmail.com). MX records are special instructions for that domain, telling other computers where to deliver emails for that domain. Our custom function looks up these MX records to see which email servers are being used. For example, if the MX records show Google servers, it's likely a Gmail or Google Workspace account. This helps us determine the email provider behind an address, even if it's not immediately obvious.
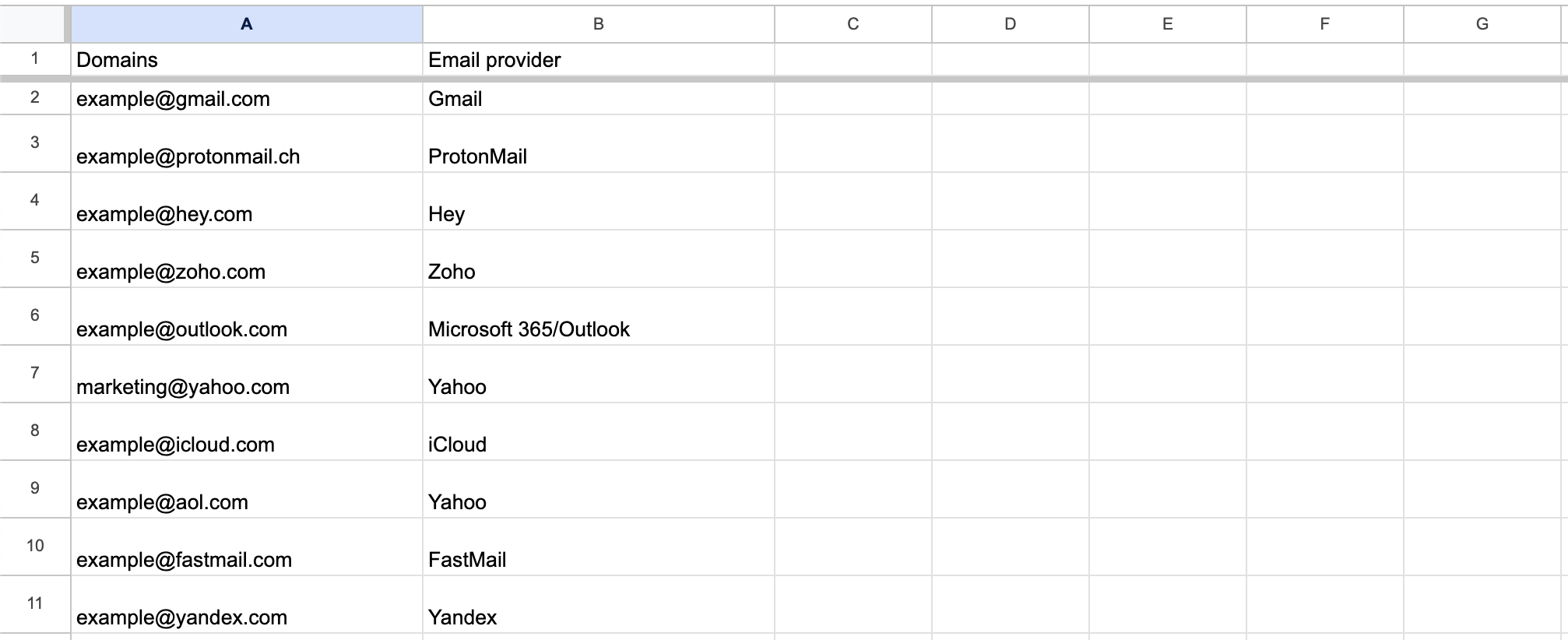
Prerequisites
This tutorial assumes you're familiar with:
3 steps to create an email provider detection function
Step 1 — Create the Apps Script project
Open Google Sheets
Click on Extensions → Apps Script
In the Apps Script editor, remove any existing code
Rename your project by clicking on "Untitled project" in the top-left corner
Step 2 — Add the custom function code
Copy and paste the following code into the Apps Script editor:
/**
* Custom function to identify email provider from MX records.
* Usage in Google Sheets:
* Single cell: =GETEMAILPROVIDER(A1)
* Range of cells: =GETEMAILPROVIDER(A1:A10)
*
* @param {string|string[][]} email The email address(es) to analyze
* @customfunction
*/
function GETEMAILPROVIDER(email) {
if (Array.isArray(email)) {
return email.map(function(row) {
return Array.isArray(row) ? row.map(getEmailProvider) : getEmailProvider(row);
});
}
if (!email || typeof email !== 'string' || email.indexOf('@') === -1) {
return 'Invalid Input';
}
var domain = email.split('@')[1].toLowerCase();
var domainProviderMap = {
'gmail.com': 'Gmail',
'outlook.com': 'Microsoft 365/Outlook',
'hotmail.com': 'Microsoft 365/Outlook',
'live.com': 'Microsoft 365/Outlook',
'yahoo.com': 'Yahoo',
'aol.com': 'Yahoo',
'icloud.com': 'iCloud',
'zoho.com': 'Zoho',
'protonmail.com': 'ProtonMail',
'pm.me': 'ProtonMail',
'mail.ru': 'Mail.ru',
'gmx.net': 'GMX',
'gmx.com': 'GMX',
'hey.com': 'Hey',
'qq.com': 'Tencent'
};
if (domainProviderMap[domain]) {
return domainProviderMap[domain];
}
// MX record lookup
try {
var url = 'https://dns.google/resolve?name=' + domain + '&type=MX';
var response = UrlFetchApp.fetch(url);
var result = JSON.parse(response.getContentText());
if (!result.Answer) {
return 'No MX Records';
}
var mxRecords = result.Answer
.map(function(record) {
return record.data.toLowerCase();
})
.join(' ');
var mxProviderMap = {
'google': 'Google Workspace',
'outlook': 'Microsoft 365/Outlook',
'microsoft': 'Microsoft 365/Outlook',
'yahoodns': 'Yahoo',
'mail.icloud': 'iCloud',
'zoho': 'Zoho',
'proton': 'ProtonMail',
'messagingengine': 'FastMail',
'emailsrvr': 'Rackspace',
'mx.yandex': 'Yandex',
'secureserver.net': 'GoDaddy',
'hostinger': 'Hostinger',
'ovh.net': 'OVH',
'ionos': 'IONOS',
'privateemail': 'Namecheap',
'amazonaws.com': 'Amazon WorkMail',
'mailgun': 'Mailgun',
'sendgrid': 'SendGrid',
'your-server.de': 'Hetzner',
'dreamhost': 'DreamHost',
'postmarkapp': 'Postmark',
'mailjet': 'Mailjet',
'bluehost': 'Bluehost',
'hostgator': 'HostGator'
};
for (var provider in mxProviderMap) {
if (mxRecords.indexOf(provider) !== -1) {
return mxProviderMap[provider];
}
}
return "Other";
} catch (error) {
console.error('Error during MX lookup:', error);
return 'Lookup Failed';
}
}
Save the script by clicking on the disk icon or pressing Ctrl + S
(⌘ + S
on Mac).
Note
To add support for additional email providers, insert a new if statement following the same pattern. For example, to add support for a provider that uses 'example.com' in its MX records, add: if (mxString.includes('example.com')) { return 'Example Provider'; }
Step 3 — Use the function in your spreadsheetReturn to your Google Sheets spreadsheet
In any cell, type =GETEMAILPROVIDER(
and select a cell containing an email address (for example, =GETEMAILPROVIDER(A2)
). The function works exactly like built-in Google Sheets functions such as UPPER
or TRIM
.
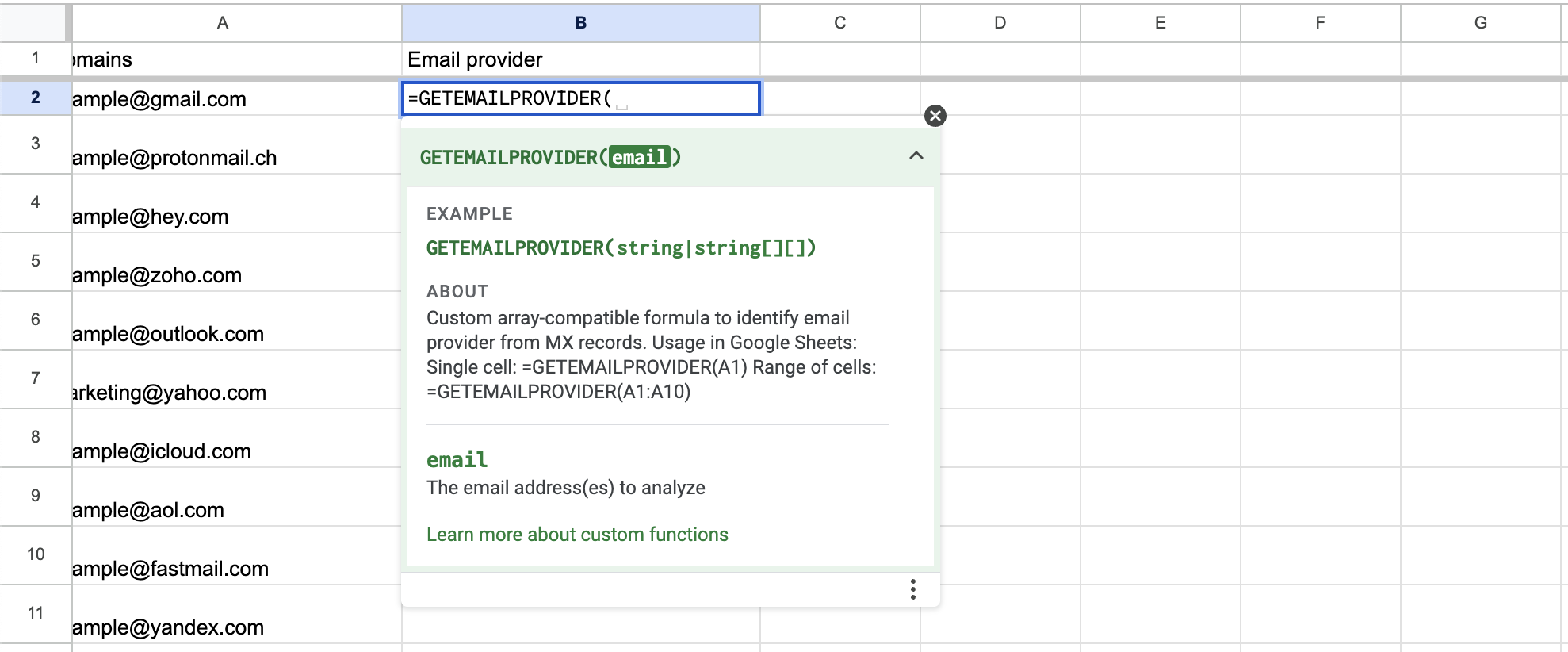
Note
You can analyze multiple email addresses at once by selecting a range of cells. For example, if your email addresses are in cells A2:A10
, you can use =GETEMAILPROVIDER(A2:A10
) to identify the provider for all emails in that range simultaneously
Rate Limits
Google's DNS API has rate limits. Apps Script's UrlFetch API also has rate limits of 20,000 per day for gmail accounts and 100,000 per day for Workspace accounts.
If you're analyzing many email addresses, spread out your requests over time or implement caching to avoid hitting these limits.
Conclusion
Return to your Google Sheets spreadsheet
In any cell, type =GETEMAILPROVIDER(
and select a cell containing an email address (for example, =GETEMAILPROVIDER(A2)
). The function works exactly like built-in Google Sheets functions such as UPPER
or TRIM
.
Note
You can analyze multiple email addresses at once by selecting a range of cells. For example, if your email addresses are in cells A2:A10
, you can use =GETEMAILPROVIDER(A2:A10
) to identify the provider for all emails in that range simultaneously
Rate Limits
Google's DNS API has rate limits. Apps Script's UrlFetch API also has rate limits of 20,000 per day for gmail accounts and 100,000 per day for Workspace accounts.
If you're analyzing many email addresses, spread out your requests over time or implement caching to avoid hitting these limits.
In this tutorial, I showed you how to create a custom function that can automatically identify email providers by analyzing MX records. This can be incredibly useful for segmenting email lists, analyzing customer data, or just organizing your contacts.
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
By subscribing, you agree to our Privacy Policy and Terms of Service