Custom functions for Google Sheets using Apps Script
As someone who frequently works with Google Sheets, I've found that custom functions are very useful for automating repetitive tasks.
What Are Custom Functions?
While Google Sheets offers powerful built-in functions like SUM
, AVERAGE
, and COUNT
, you can extend its capabilities by writing your own functions using Apps Script. These are called custom functions. Once you create these functions, you can use them in your spreadsheet formulas just like native functions.
Prerequisites
This tutorial assumes that you are familiar with the following concepts:
Writing your first custom function
Let's start with a simple example: creating a function that returns your lucky number. While this may seem basic, it introduces key concepts we'll build upon. Before coding, let's outline a few details:
Purpose: Return a predetermined lucky number
Function Name: Pick a descriptive name (eg.
MYLUCKYNUMBER
)Input Parameters: None required
Output: A numerical value (your lucky number).
I think the number 3 brings me luck so here is a function that returns my lucky number.
function MYLUCKYNUMBER () {
return 3;
}
Here is a screenshot of the above function in the Apps Script editor.
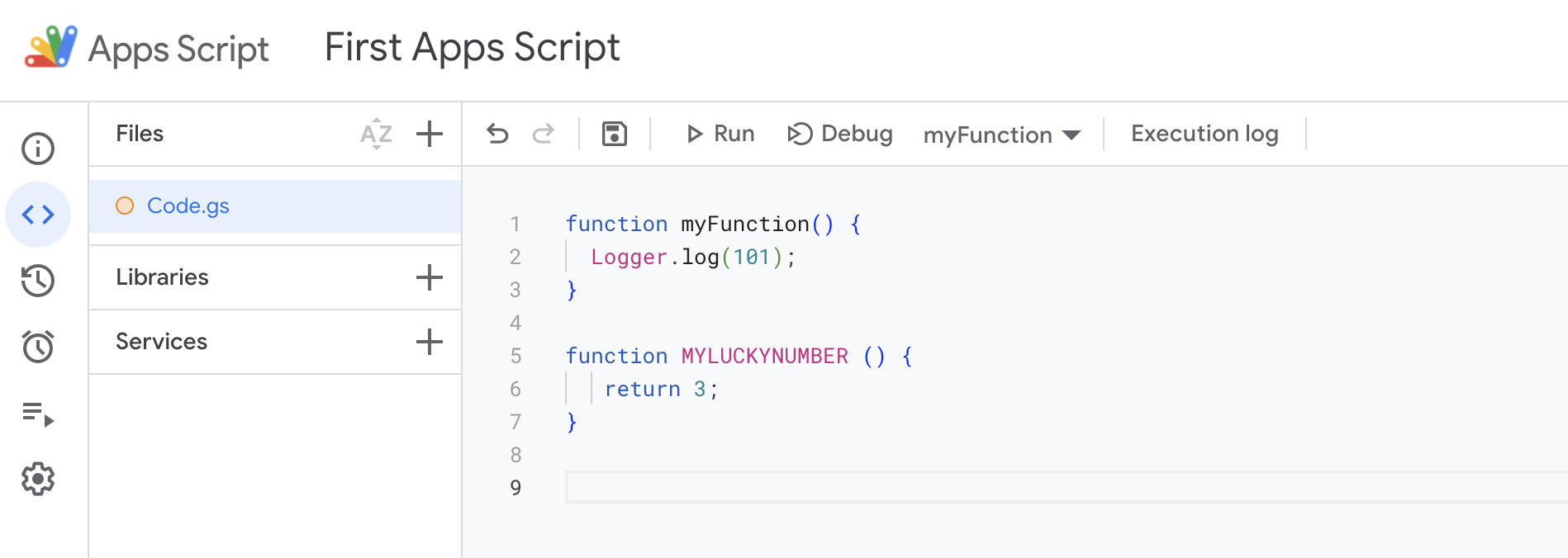
You can now use this custom function in a Google Sheets formula just like any other function.
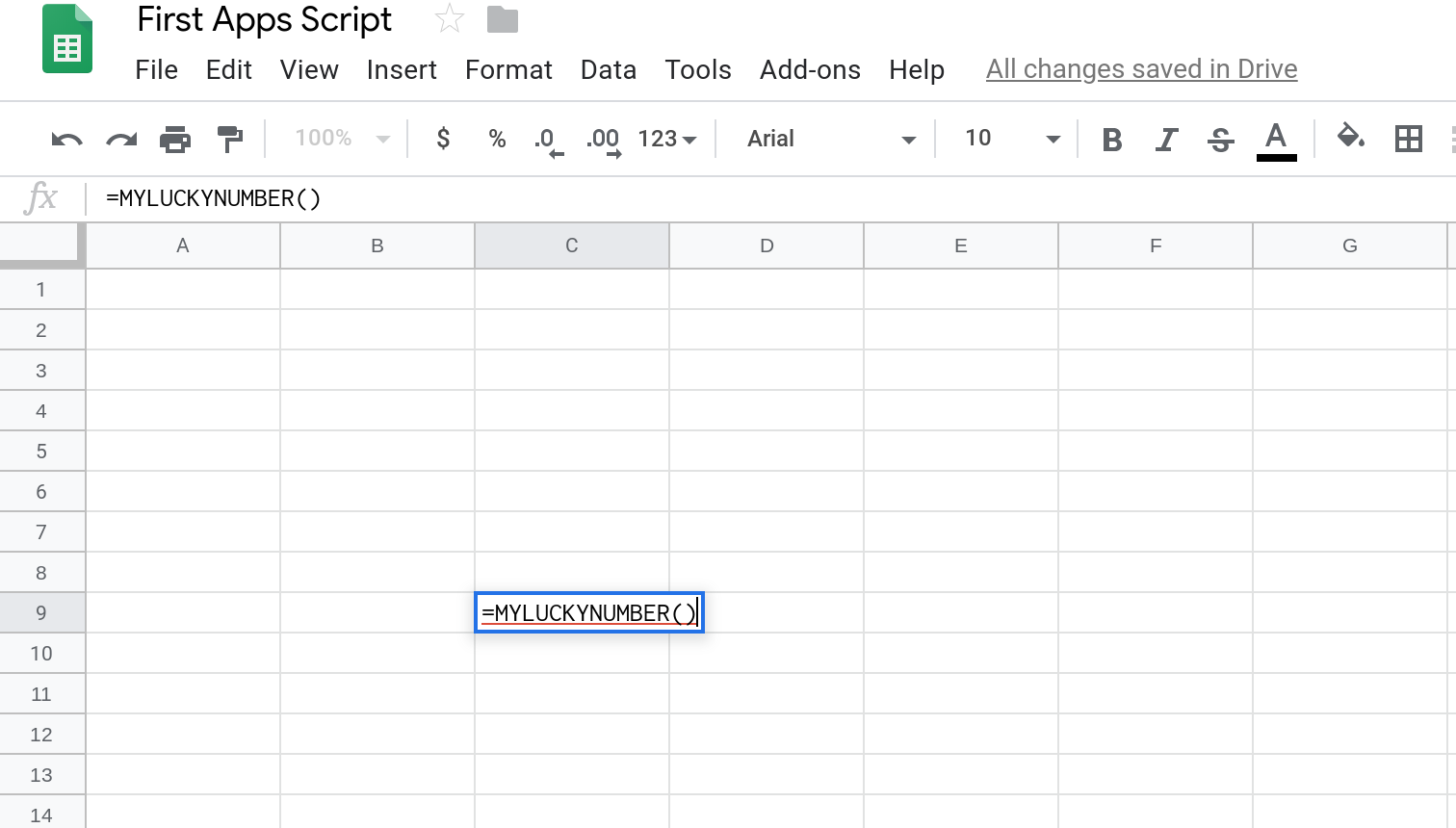
You should see your lucky number displayed in the cell where you entered the formula.
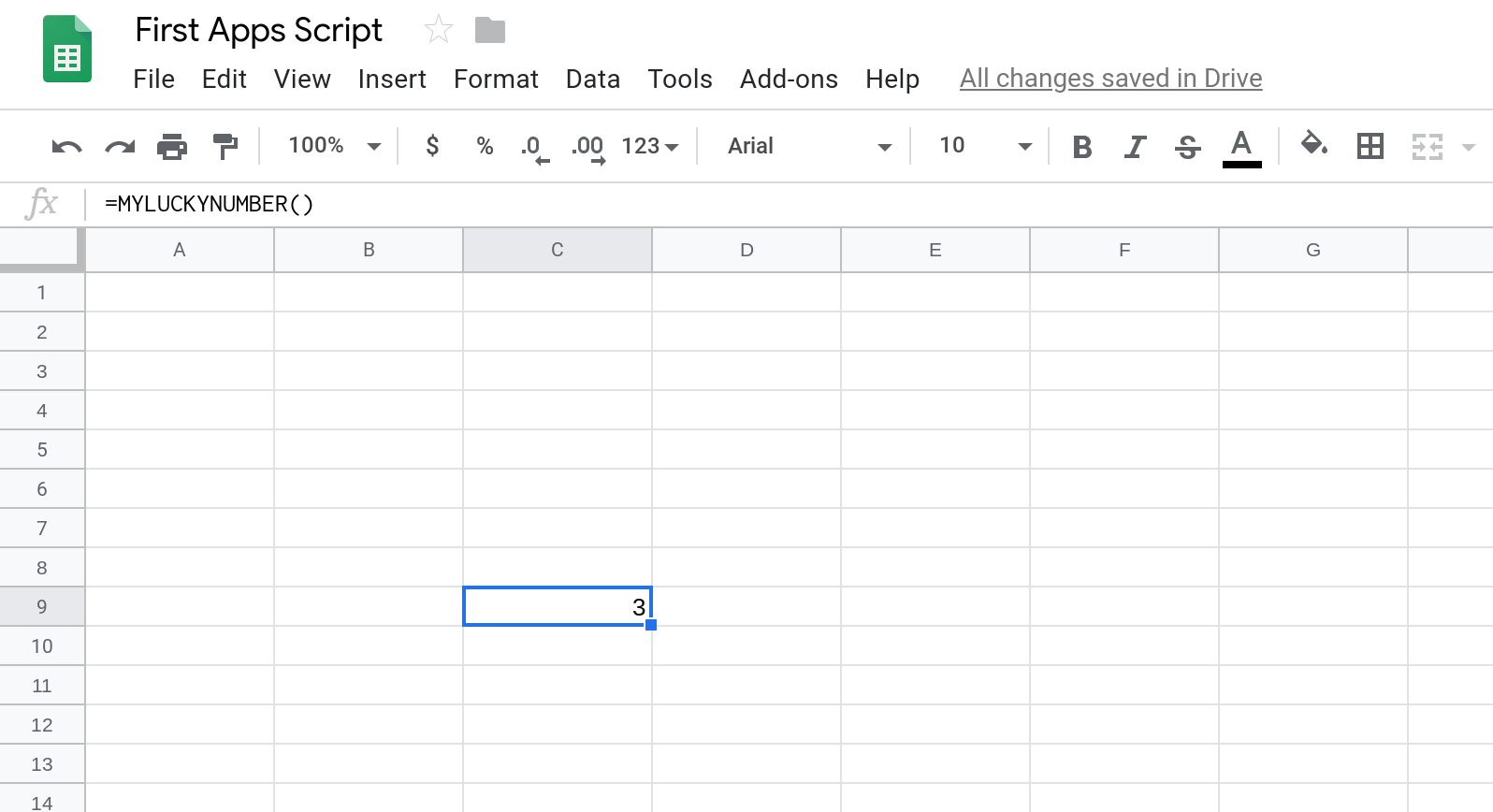
Learn more
You can learn more about custom functions in Google Sheets by reading the following tutorials:
The following tutorials are examples of custom functions that you can create:
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
By subscribing, you agree to our Privacy Policy and Terms of Service