How to automate importing Kit (ConvertKit) Subscribers to Google Sheets
Are you managing an email list with Kit (formerly known as ConvertKit) and need to access or analyze your subscriber data in Google Sheets? In this tutorial, I'll walk you through building a simple automation that imports all your Kit subscribers directly into Google Sheets. This project is perfect for content creators, small businesses, or marketers who want to maintain a current subscriber list in their spreadsheets without manual exports. Whether you need to analyze subscriber growth, or create custom marketing reports, this automation will save you time and ensure your data is always up-to-date.
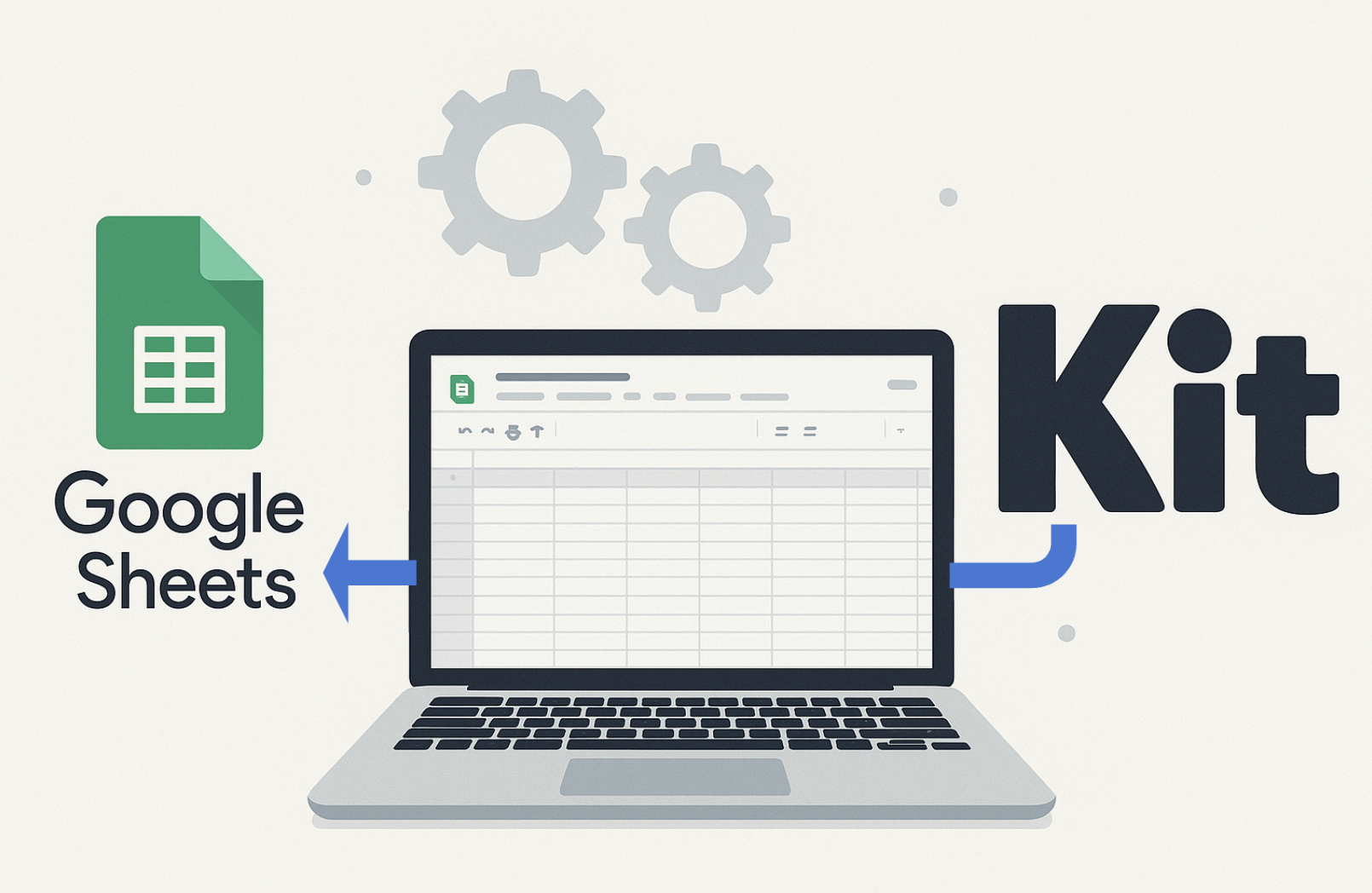
Prerequisites
This tutorial assumes the following prerequisites:
A Kit account
Basic understanding of Google Sheets
Familiarity with Apps Script fundamentals
Knowledge of how to create custom menus in Google Sheets
6 steps to implement the Kit subscriber import automation
Step 1 — Create a new Google Sheet
First, let's create a new Google Sheet that will store our Kit subscriber data:
Go to sheets.new to create a new Google Sheet
Rename the spreadsheet to something descriptive like "Kit Subscribers"
Rename "Sheet1" to "Subscribers" by right-clicking on the sheet tab at the bottom and selecting "Rename"
In cell A1, type "Email Address" as the header for our data
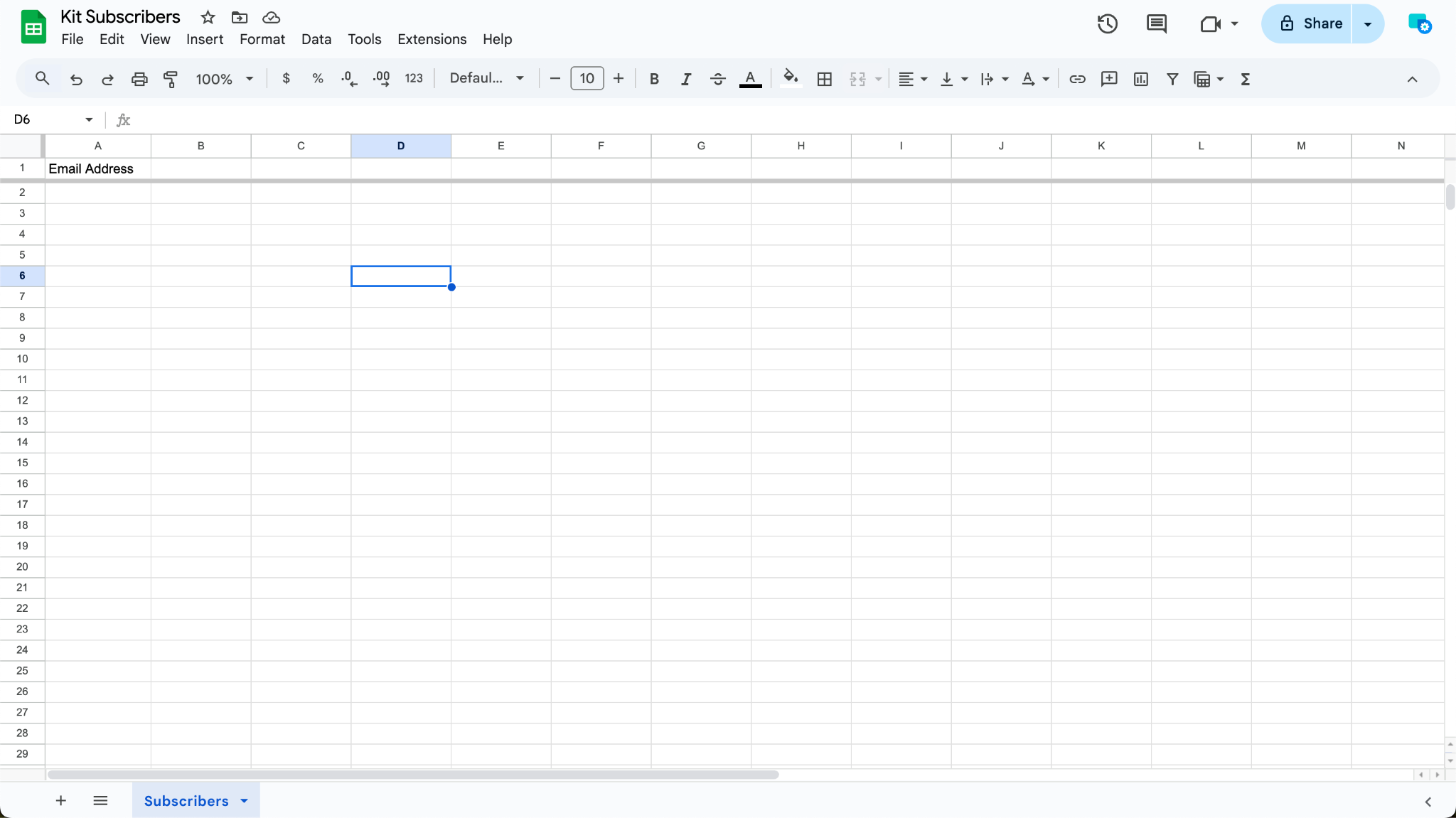
Step 2 — Set up your Apps Script project
Now, let's create the Apps Script project that will handle the integration with Kit:
In your Google Sheet, click on Extensions → Apps Script
Rename the project to "Kit Integration" by clicking on the current project name at the top
Delete any default code in the editor
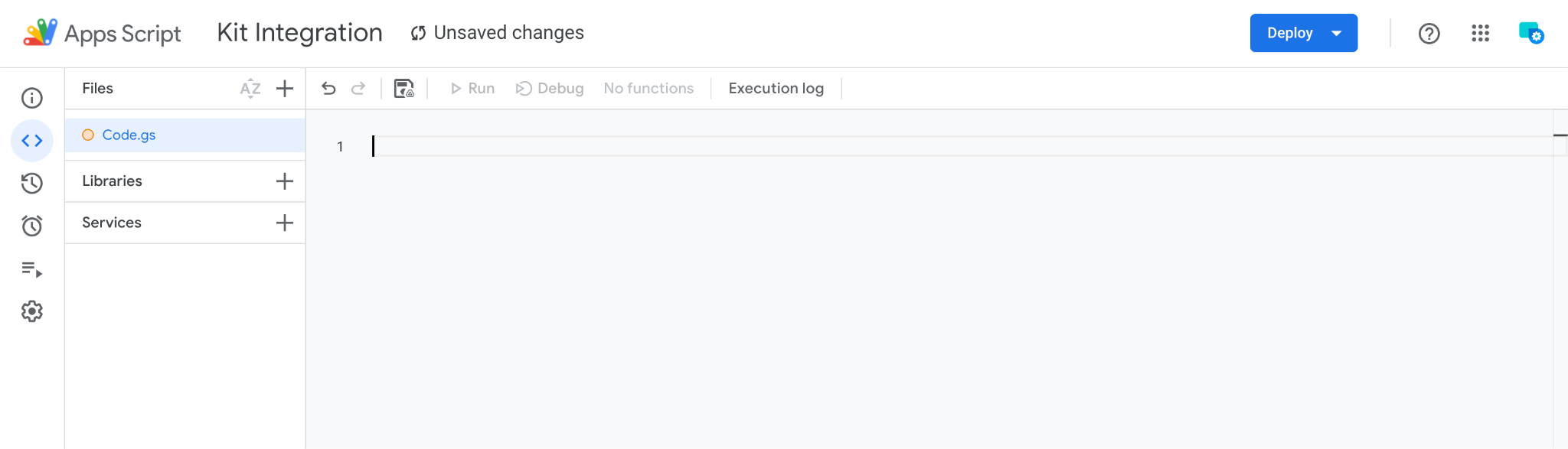
Step 3 — Get your Kit API key
To access the Kit API, you'll need an API key:
Log in to your Kit account
Go to Account Settings → Developer Settings or navigate directly to https://app.kit.com/account_settings/developer_settings
Copy your API V4 Key (or create one if you don't have one yet)
Note
We will be using Kit's V4 API so make sure to create a V4 key (and not a V3 key).
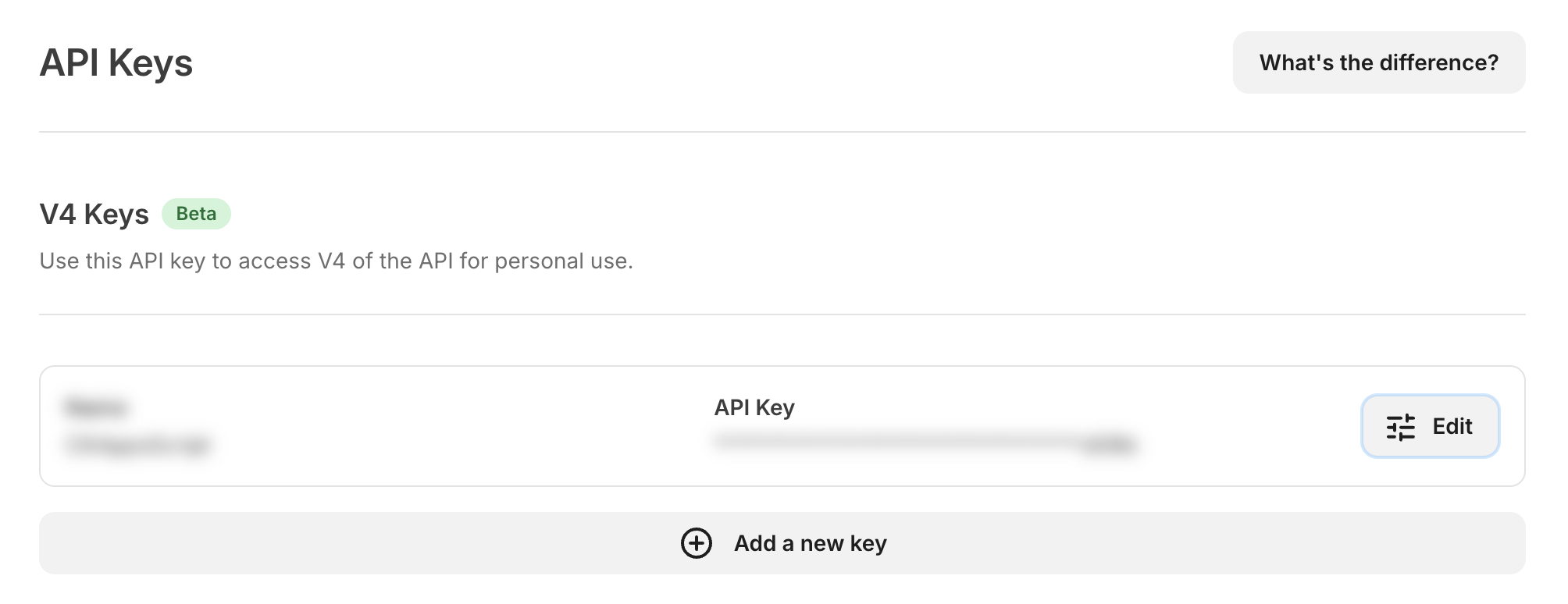
Now let's store this API key in your Apps Script project:
In the Apps Script editor, click on Project Settings (the gear icon)
Scroll down to Script Properties
Click Add Script Property
For the property name, enter
CK_API_KEY
For the value, paste your Kit API key
Click Save script properties
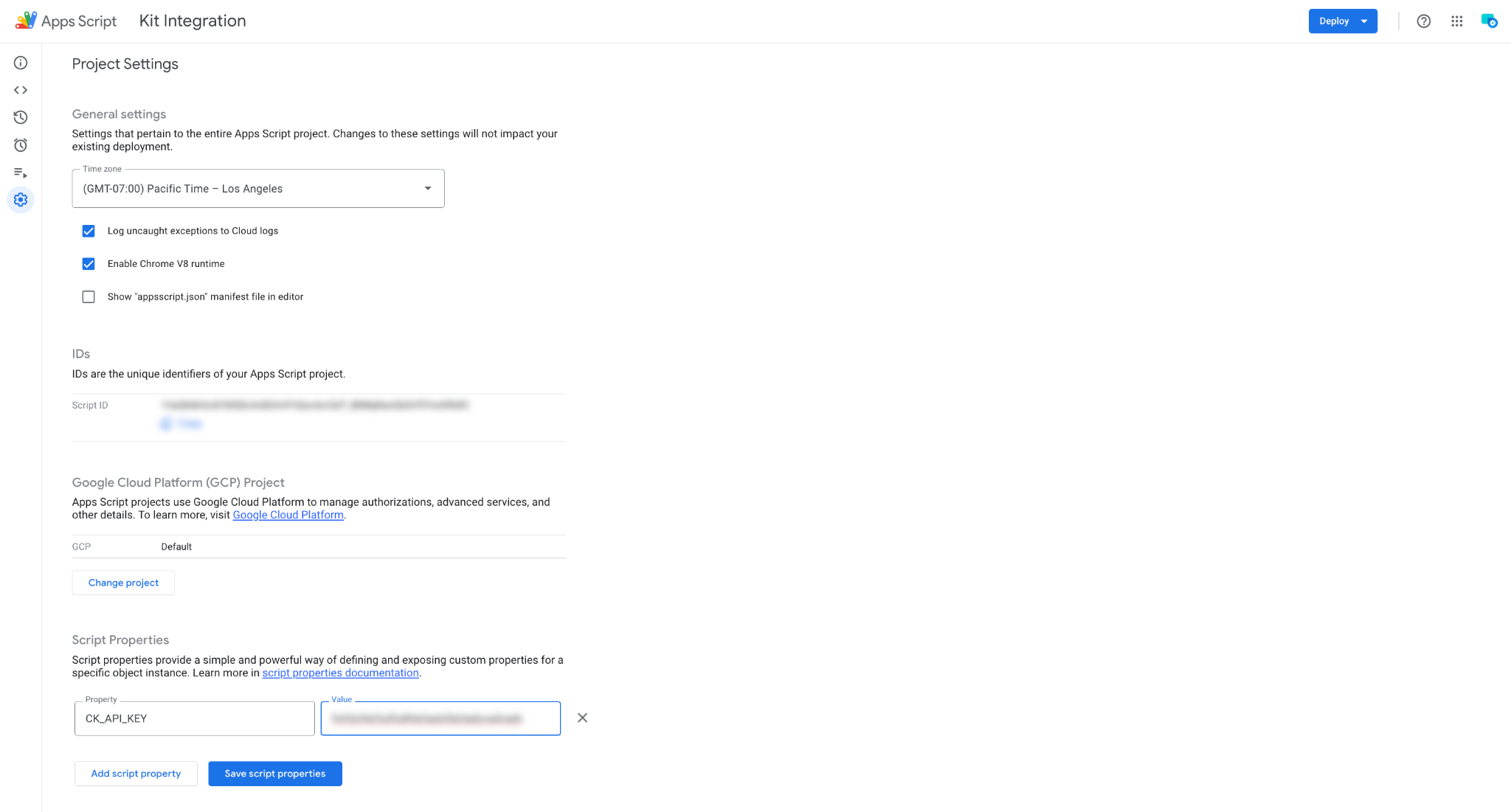
Step 4 — Write the code to fetch subscribers
Now, let's write the code that will connect to the Kit API and import your subscribers. Copy and paste the following code into your Apps Script editor:
//@OnlyCurrentDoc
/**
* Fetches subscriber email addresses from Kit API v4 and imports them
* into a single column in the bound Google Sheet.
*/
function importKitSubscribers() {
//Configure API Key and Sheet Details
const apiKey = PropertiesService.getScriptProperties().getProperty("CK_API_KEY");
if(!apiKey) {
Logger.log("Error: Kit API Key not found.");
SpreadsheetApp.getActive().toast("Error: Kit API Key not found.");
return;
}
const sheetName = "Subscribers"; // Replace with the sheet name for storing emails
//Get the Spreadsheet and Sheet
const ss = SpreadsheetApp.getActiveSpreadsheet();
const sheet = ss.getSheetByName("Subscribers");
sheet.clear();
sheet.appendRow(["Email Address"]);
//Set up API Request
const baseUrl = "https://api.kit.com/v4/subscribers";
const headers = {
"X-Kit-Api-Key": `${apiKey}`,
"Accept": "application/json"
};
let hasNextPage = true;
let afterCursor = null;
//Fetch and Process Subscribers
while (hasNextPage) {
let url = `${baseUrl}?per_page=500`; // Fetch up to 500 per page
if (afterCursor) {
url += `&after=${afterCursor}`;
}
const options = {
"method": "get",
"headers": headers
};
// Make the API request:
const response = UrlFetchApp.fetch(url, options);
const data = JSON.parse(response.getContentText());
// Extract email addresses:
const subscribers = data.subscribers;
const emailAddresses = subscribers.map(subscriber => [subscriber.email_address]);
// Append data to the sheet:
sheet.getRange(sheet.getLastRow() + 1, 1, emailAddresses.length, 1).setValues(emailAddresses);
// Check for pagination:
hasNextPage = data.pagination.has_next_page;
afterCursor = data.pagination.end_cursor;
}
SpreadsheetApp.getActive().toast("Subscribers imported successfully.");
}
Step 5 — Create a custom menu
Next, let's add a custom menu to make it easy for you to run the import function. Add the following code to your script:
/**
* Creates a custom menu in the Google Sheet when the spreadsheet is opened.
*/
function onOpen() {
const ui = SpreadsheetApp.getUi();
ui.createMenu('📧 Kit')
.addItem('Import Subscribers', 'importKitSubscribers')
.addToUi();
}
Now save your script by pressing Ctrl+S (or Cmd+S on Mac).
Step 6 — Test your integration
Let's test our integration to make sure everything works correctly:
Return to your Google Sheet
Refresh the page to see the new "Kit" menu appear
Click on Kit → Import Subscribers
The first time you run the script, you'll need to authorize it.
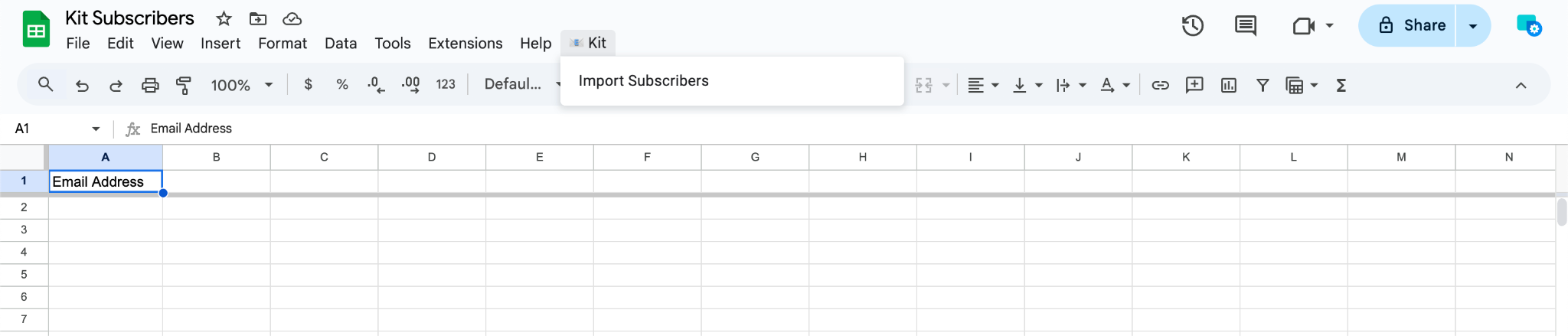
After running the script, you should see your Kit subscribers' email addresses populated in column A, starting from row 2:
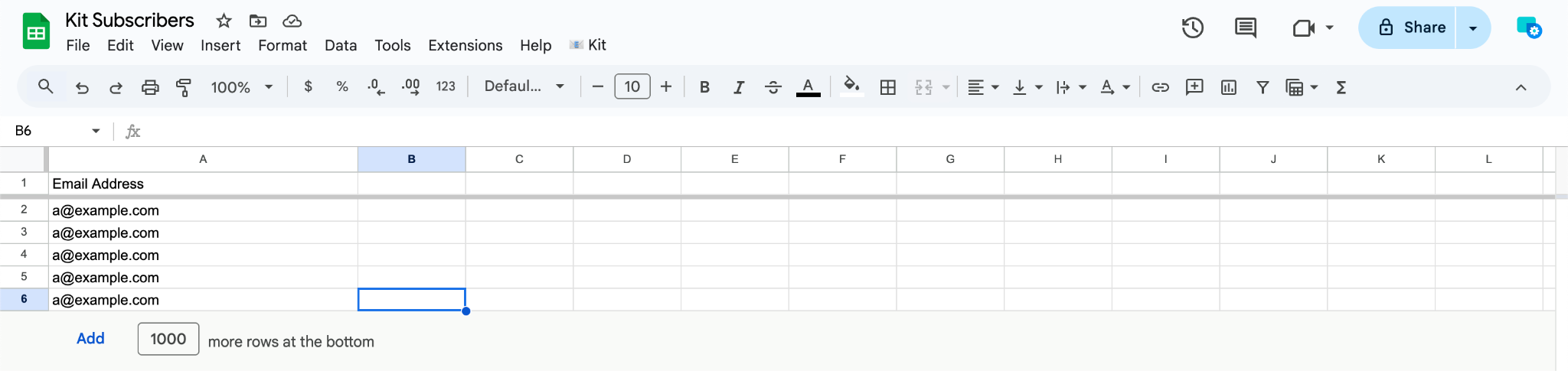
Conclusion
In this tutorial, you've learned how to create an integration between Kit and Google Sheets using Apps Script. This automation allows you to easily import all your Kit subscribers into a Google Sheet with just a single click, saving you time and ensuring your data is up-to-date.
I also showed you how to set up the Apps Script project, configure your API key, write code to fetch and process the Kit API data, handle pagination, and create a custom menu for easy access. You now have a powerful tool that you can use whenever you need to analyze or work with your subscriber list in Google Sheets.
Thank you for following along with this tutorial. I hope this integration helps streamline your email marketing workflows.
Future work
Here are some ways you could enhance this integration:
Import additional subscriber data: Modify the code to import more subscriber data beyond just email addresses, such as names, tags, and subscription dates. This would involve adjusting the API request and adding more columns to your sheet.
Create an automatic trigger: Set up a time-based trigger to run the import automatically on a schedule (e.g., daily or weekly) so your sheet is always up to date without manual intervention.
Add filtering options: Enhance the script to allow filtering subscribers by tags, subscription status, or date ranges. This would make it easier to analyze specific segments of your audience.
Build a two-way sync: Extend the functionality to not just import subscribers but also update Kit based on changes made in the sheet. For example, you could add subscribers to specific tags based on values in your spreadsheet.
Create a dashboard: Use the imported data to build a subscriber analytics dashboard in Google Sheets with charts and metrics showing growth trends, engagement patterns, and other insights about your email list.
How was this tutorial?
Your feedback helps me create better content
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
By subscribing, you agree to our Privacy Policy and Terms of Service