How to Export Google Docs to PDF Using Apps Script (with Tab Support)
Exporting a Google Doc to a PDF is a very common use case in various automation workflows. Ever since Google launched support for "Tabs" in Google Docs, a cover page automatically gets added to the exported PDF for every tab in the Google Doc. A Google Doc containing a single page becomes two pages when you export it to a PDF: the first page will be a cover page with the Tab's name and the second page will be the actual contents of that tab.
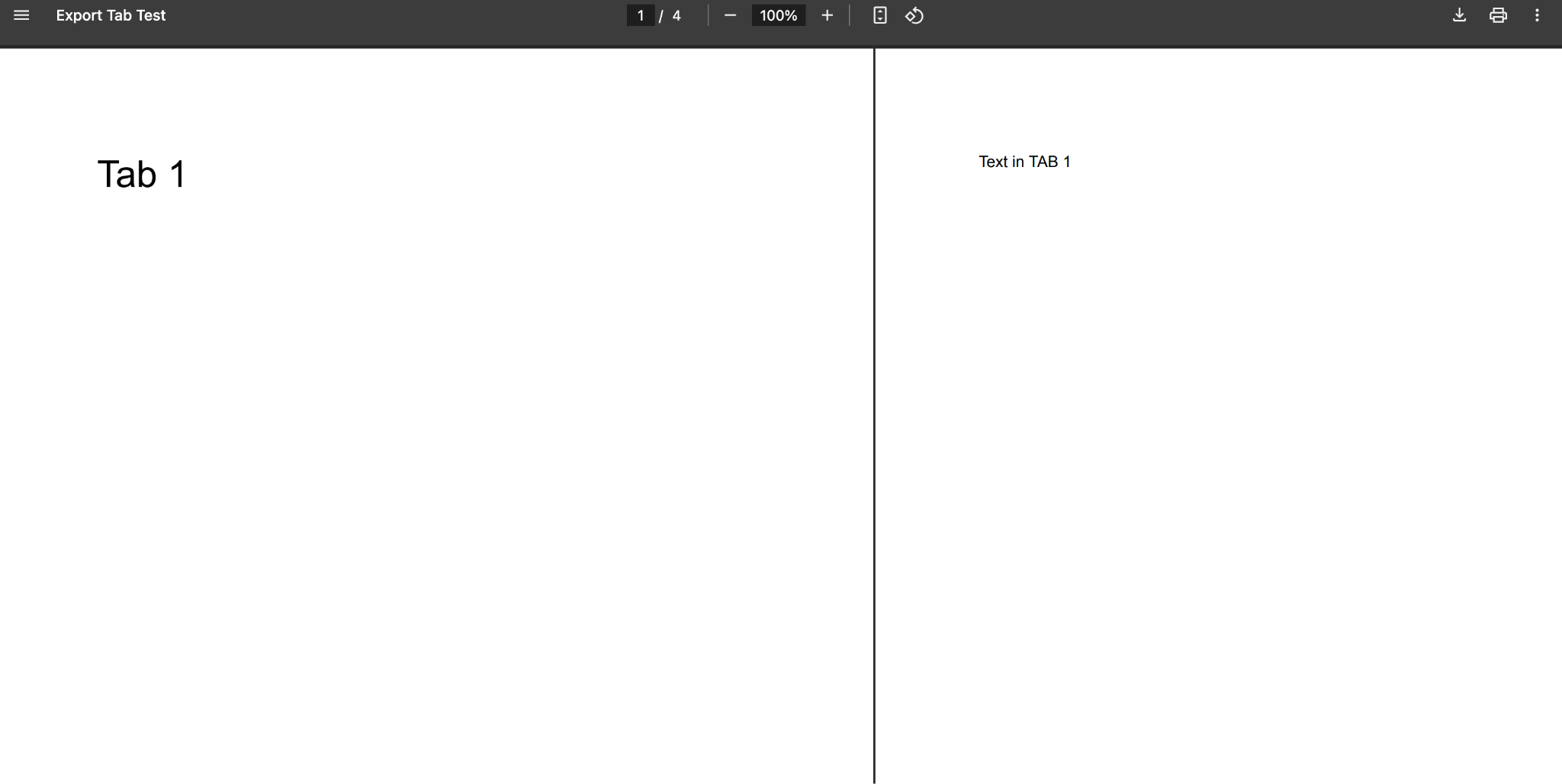
This can be frustrating - imagine exporting an invoice to PDF only to see a random cover page called "Tab 1" inserted into your PDF!
In this tutorial, I will show you how to export Docs to PDF with or without these cover pages. You'll find this tutorial particularly useful for use cases such as:
Automated report generation or other workflow automation scenarios
Batch processing of documents
Creating PDF archives of your documents
Apps Script function to export Google Docs to PDF
Here's an Apps Script function that exports a Google Doc to a PDF. It can export entire documents or a specific tab.
/**
* Exports a Google Doc to PDF, optionally specifying a tab to export.
* @param {string} docId The ID of the Google Doc.
* @param {object} [options] Optional parameters.
* @param {string} [options.fileName] The name of the exported PDF file. Defaults to the Doc's name.
* @param {number} [options.tabIndex] The index of the tab to export (1-based).
* @param {string} [options.tabId] The ID of the tab to export.
* @returns {string} The ID of the newly created PDF file in Google Drive.
* @throws {Error} If docId is missing, tabIndex is out of bounds, or tabId is invalid.
*/
function exportGoogleDocToPDF(docId, options = {}) {
if (!docId) {
throw new Error("docId is required.");
}
const doc = DocumentApp.openById(docId);
let url = `https://docs.google.com/document/d/${doc.getId()}/export?format=pdf`;
// Export tab
if(options.hasOwnProperty("tabIndex") || options.hasOwnProperty("tabId")) {
const tabs = doc.getTabs();
let tabId = null;
if(options.hasOwnProperty("tabId")) {
tabId = options["tabId"];
const tabExists = tabs.some(tab => tab.getId() === options.tabId);
if (!tabExists) {
throw new Error("Invalid tabId provided.");
}
} else if(options.hasOwnProperty("tabIndex")) {
if(tabs.length < options["tabIndex"]) {
throw new Error("Tab index is out of bounds.");
}
tabId = tabs[options["tabIndex"] - 1].getId();
}
url = url + `&tab=${tabId}`;
}
const params = {
headers: {
"Authorization": 'Bearer ' + ScriptApp.getOAuthToken()
}
};
const response = UrlFetchApp.fetch(url, params);
const fileName = options.hasOwnProperty("fileName") ? options["fileName"] : doc.getName();
const blob = response.getBlob();
blob.setName(fileName);
const exportedFile = DriveApp.createFile(blob)
return exportedFile.getId();
}
How to Use ItBasic Export
The simplest way to use the function is to export an entire document:
const docId = "<DOC_ID>"; // Replace with your Google Doc's ID
const pdfId = exportGoogleDocToPDF(docId);
If you do not specify a tab, all the tabs in the document will be exported. Also, for each tab, the exported PDF file will contain a cover page with its name that precedes its contents.
For example, consider the following Google Doc that has two tabs:
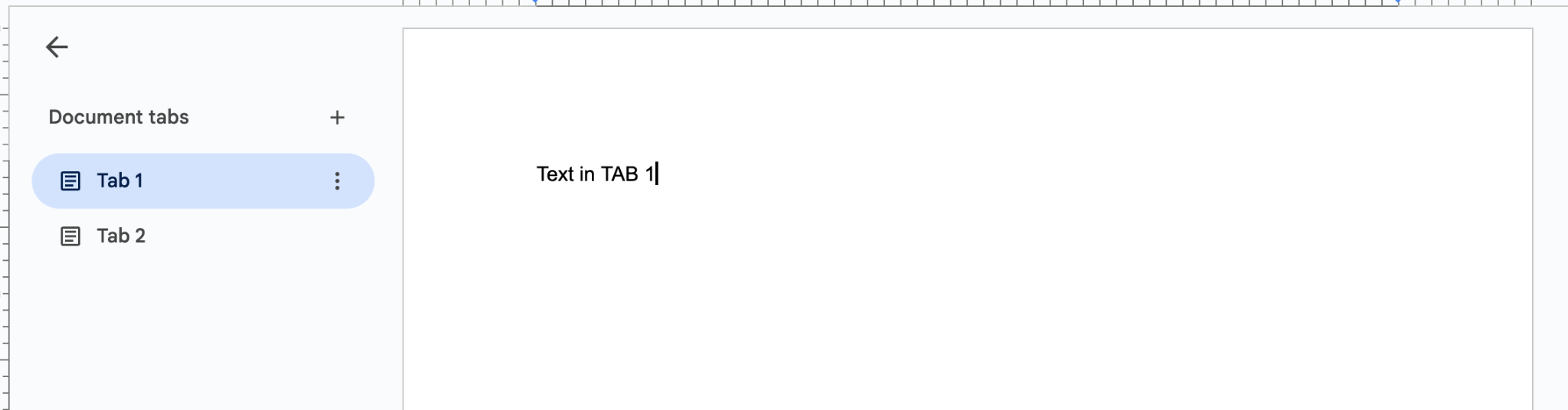
The exported PDF file will have 4 pages:
A cover page for Tab 1
Tab 1's contents
A cover page for Tab 2
Tab 2's contents
If you specify a tab to export (by specifying either its index or its ID), only its contents will be exported. In this case, a cover sheet will not be included.
Export with Custom Filenameconst docId = "<DOC_ID>"; // Replace with your Google Doc's ID
const pdfId = exportGoogleDocToPDF(docId, {
fileName: 'Monthly-Report-Jan-2024.pdf'
});
Export Specific Tab by Indexconst docId = "<DOC_ID>"; // Replace with your Google Doc's ID
const pdfId = exportGoogleDocToPDF(docId, {
tabIndex: 1 // Exports first tab
});
Export Specific Tab by IDconst docId = "<DOC_ID>"; // Replace with your Google Doc's ID
const pdfId = exportGoogleDocToPDF(docId, {
tabId: 'tab_id_12345'
});
Important NotesThe docId
can be found in the URL of your Google Doc:
https://docs.google.com/document/d/DOC_ID/edit
The tabId can also be found in the URL of your Google Doc:
https://docs.google.com/document/d/DOC_ID/edit?tab=TAB_ID
Tab indices are 1-based (first tab is index 1)
The function returns the ID of the newly created PDF in your Google Drive
The default filename will match the original document's name if not specified
The function preserves the original document and creates a new PDF file
Remember to handle the returned PDF ID according to your use case - you might want to move the PDF to a specific folder or share it with others.
How was this tutorial?
Your feedback helps me create better content
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
Exclusive Google Sheets automation tutorials and hands-on exercises
Ready-to-use scripts and templates that transform hours of manual work into seconds
Email updates with new automation tips and time-saving workflows
By subscribing, you agree to our Privacy Policy and Terms of Service
const docId = "<DOC_ID>"; // Replace with your Google Doc's ID
const pdfId = exportGoogleDocToPDF(docId, {
fileName: 'Monthly-Report-Jan-2024.pdf'
});
const docId = "<DOC_ID>"; // Replace with your Google Doc's ID
const pdfId = exportGoogleDocToPDF(docId, {
tabIndex: 1 // Exports first tab
});
Export Specific Tab by IDconst docId = "<DOC_ID>"; // Replace with your Google Doc's ID
const pdfId = exportGoogleDocToPDF(docId, {
tabId: 'tab_id_12345'
});
Important NotesThe docId
can be found in the URL of your Google Doc:
https://docs.google.com/document/d/DOC_ID/edit
The tabId can also be found in the URL of your Google Doc:
https://docs.google.com/document/d/DOC_ID/edit?tab=TAB_ID
Tab indices are 1-based (first tab is index 1)
The function returns the ID of the newly created PDF in your Google Drive
The default filename will match the original document's name if not specified
The function preserves the original document and creates a new PDF file
Remember to handle the returned PDF ID according to your use case - you might want to move the PDF to a specific folder or share it with others.
How was this tutorial?
Your feedback helps me create better content
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
Exclusive Google Sheets automation tutorials and hands-on exercises
Ready-to-use scripts and templates that transform hours of manual work into seconds
Email updates with new automation tips and time-saving workflows
By subscribing, you agree to our Privacy Policy and Terms of Service
const docId = "<DOC_ID>"; // Replace with your Google Doc's ID
const pdfId = exportGoogleDocToPDF(docId, {
tabId: 'tab_id_12345'
});
Important NotesThe docId
can be found in the URL of your Google Doc:
https://docs.google.com/document/d/DOC_ID/edit
The tabId can also be found in the URL of your Google Doc:
https://docs.google.com/document/d/DOC_ID/edit?tab=TAB_ID
Tab indices are 1-based (first tab is index 1)
The function returns the ID of the newly created PDF in your Google Drive
The default filename will match the original document's name if not specified
The function preserves the original document and creates a new PDF file
The docId
can be found in the URL of your Google Doc:
https://docs.google.com/document/d/DOC_ID/edit
The tabId can also be found in the URL of your Google Doc:
https://docs.google.com/document/d/DOC_ID/edit?tab=TAB_ID
Tab indices are 1-based (first tab is index 1)
The function returns the ID of the newly created PDF in your Google Drive
The default filename will match the original document's name if not specified
The function preserves the original document and creates a new PDF file
Remember to handle the returned PDF ID according to your use case - you might want to move the PDF to a specific folder or share it with others.
How was this tutorial?
Your feedback helps me create better content
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
By subscribing, you agree to our Privacy Policy and Terms of Service