The forEach() loop in Apps Script
The Array method forEach()
is used to execute a function for every element in an array. It is also referred to as the forEach()
loop because it is a common way to loop through every element of the array to take some action on each element.
Prerequisites
This tutorial assumes that you're familiar with:
Syntaxarray.forEach(callbackFunction)
Parameters
array.forEach(callbackFunction)
callbackFunction (required):
This is the function that will be executed once for every element in the array.
The callbackFunction
can accept three arguments:
value
(required): The current value being processed.index
(optional): The index of the value in the array.array
(optional): The original array that theforEach()
method was called on.
Important
The forEach()
method will skip values in the array that are undefined.
Consider the array ['red','green',,'blue']
. The callbackFunction
will only be executed three times even though the length of the array is 4. This is because the third value in the array is undefined
and the forEach()
method will skip it.
Return value
The forEach()
method will return undefined
.
ExamplesExample 1: Log the elements of an array
Example 1.1
In the code below, the function logValue(value)
is the callback parameter in the forEach()
method. This function is executed once for every element in the array colors
and it logs that value.
function forEachEx1_1() {
var colors = ["red", "blue", "green", "black", "orange", "purple"];
colors.forEach(logValue);
}
function logValue(value) {
Logger.log(value);
}
How does the above code work?
The forEach()
method will execute the callback function once for every element in the array colors
that isn't undefined.
Note: undefined
values will be skipped by the forEach()
method.
Therefore, the function logValue()
will be called 6 times, once for every element in the array colors
.
logValue("red");
logValue("blue");
logValue("green");
logValue("black");
logValue("orange");
logValue("purple");
This results in each value being logged.
Output
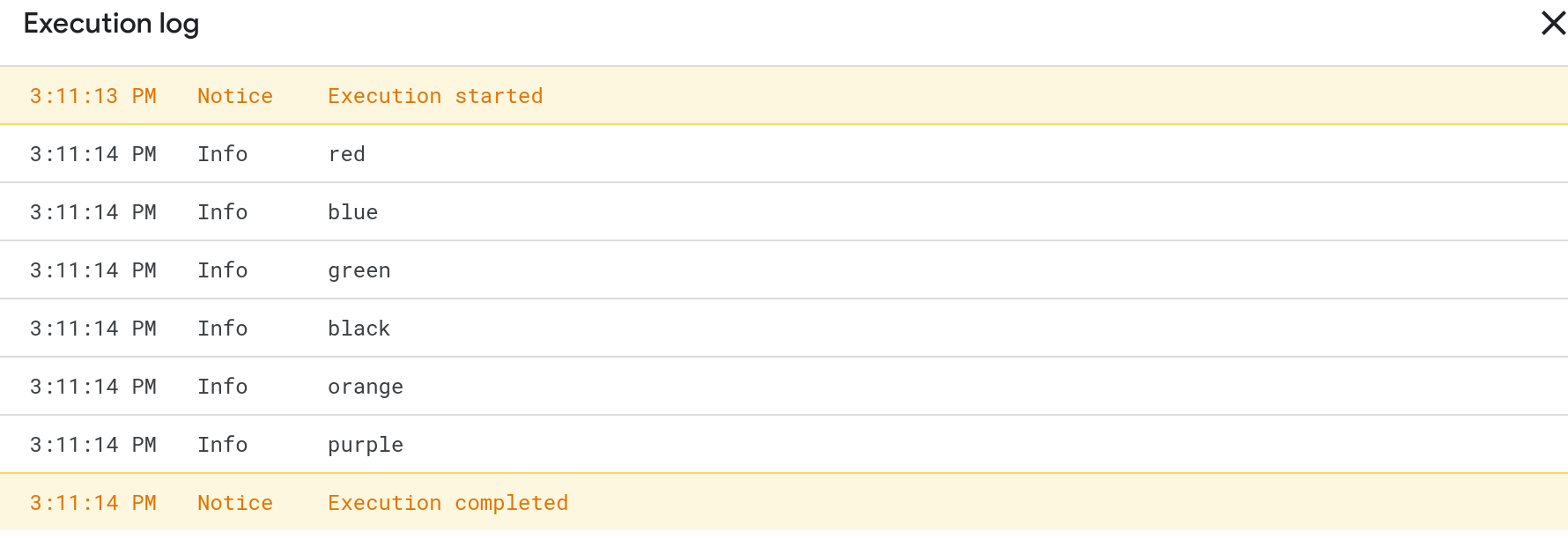
Example 1.2
Instead of defining the function logValue() separately and then passing it as a parameter, you can also define it in-line. The code below shows you how to do this.
function forEachEx1_2() {
var colors = ["red", "blue", "green", "black", "orange", "purple"];
colors.forEach(function(value) {
Logger.log(value);
});
}
Output
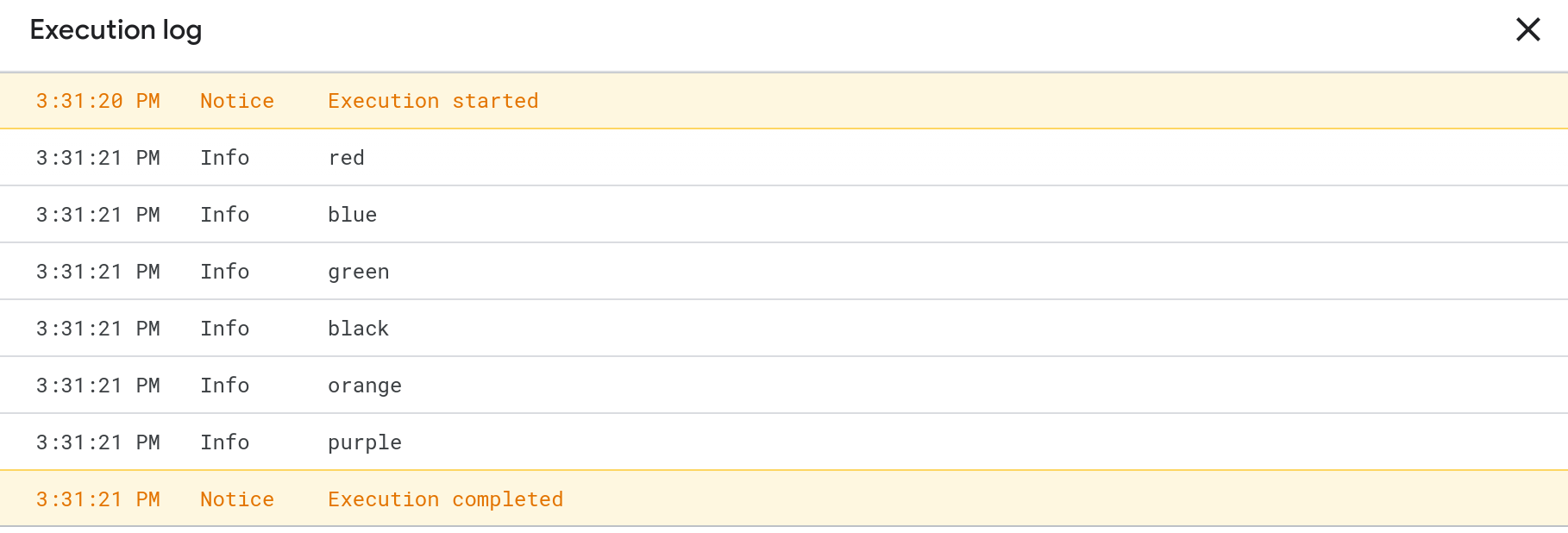
Note
Notice that there is no name for the function in the above example.
This is because, in Apps Script (which is a version of JavaScript), a function is basically a variable with a value of type function.
Consider the function addTwoNumbers()
below.
function addTwoNumbers(num1, num2) {
return num1 + num2;
}
This function can also be rewritten in the following way:
var addTwoNumbers = function(num1, num2) {
return num1 + num2;
}
Here the variable addTwoNumbers
contains a value of type function. In Apps Script, both of the above code snippets have the exact same result.
When you pass a function as a parameter to the forEach()
method, you're really passing the function value. Therefore, the following statement colors.forEach(logValue);
in Example 1.1 is the same as the code snippet below in Example 1.2
colors.forEach(function(value) {
Logger.log(value);
});
This is because logValue
is just a variable that contains the following function value:
function(value) {
Logger.log(value);
}
Example 2: Log the elements of an array along with their index
The code below logs the elements in the array colors
along with each element's index. Here, we use the required parameter of the callback function which is the value and an optional parameter which is the index. When the callback function is executed for an element, the elements value and index will be passed to it and this information is logged.
function forEachEx2() {
var colors = ["red", "blue", "green", "black", "orange", "purple"];
colors.forEach(function(value, index) {
Logger.log("The value at index " + index + " is " + value + ".");
});
}
Output
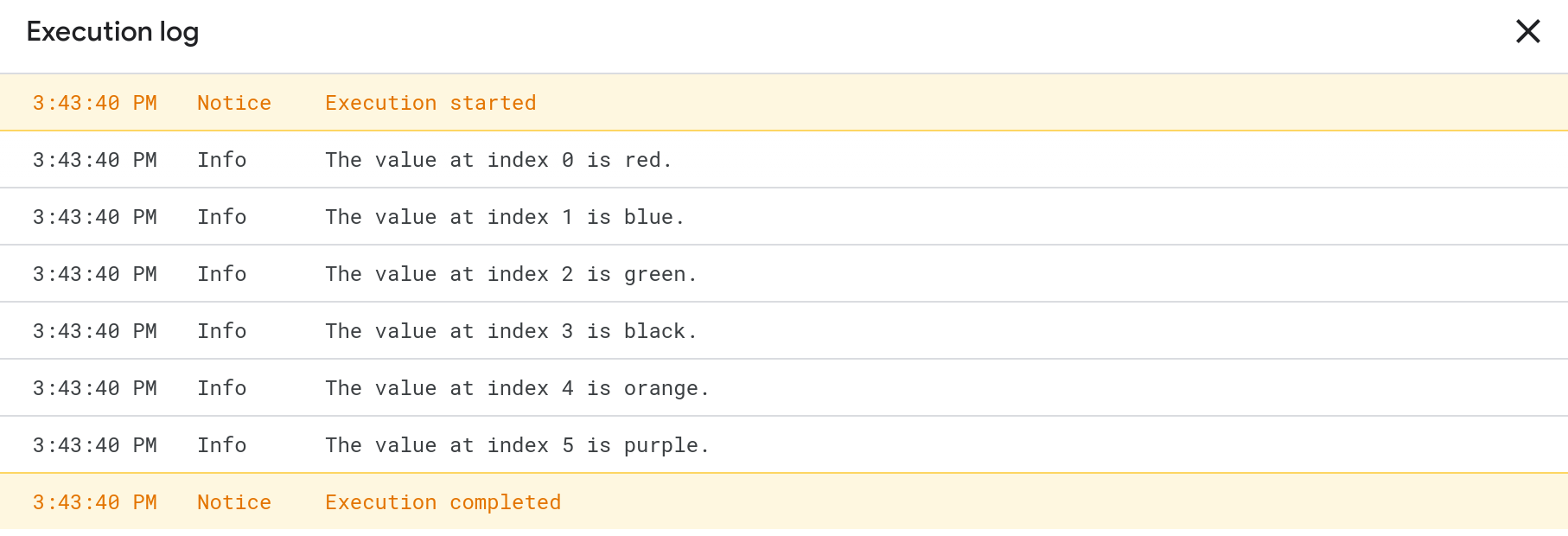
Example 3: Access the array inside the callback function
In the code below, we use the required parameter of the callback function which is the value
and two optional parameters:
index
: This is the index of the value in the array.array
: The original array itself.
In each iteration, the code will log the value, its index and the original array.
function forEachEx3() {
var colors = ["red", "blue", "green", "black", "orange", "purple"];
colors.forEach(function(value, index, array) {
Logger.log("The value at index " + index + " is " + value + ".");
Logger.log(array); //Logs the original array
});
}
Output
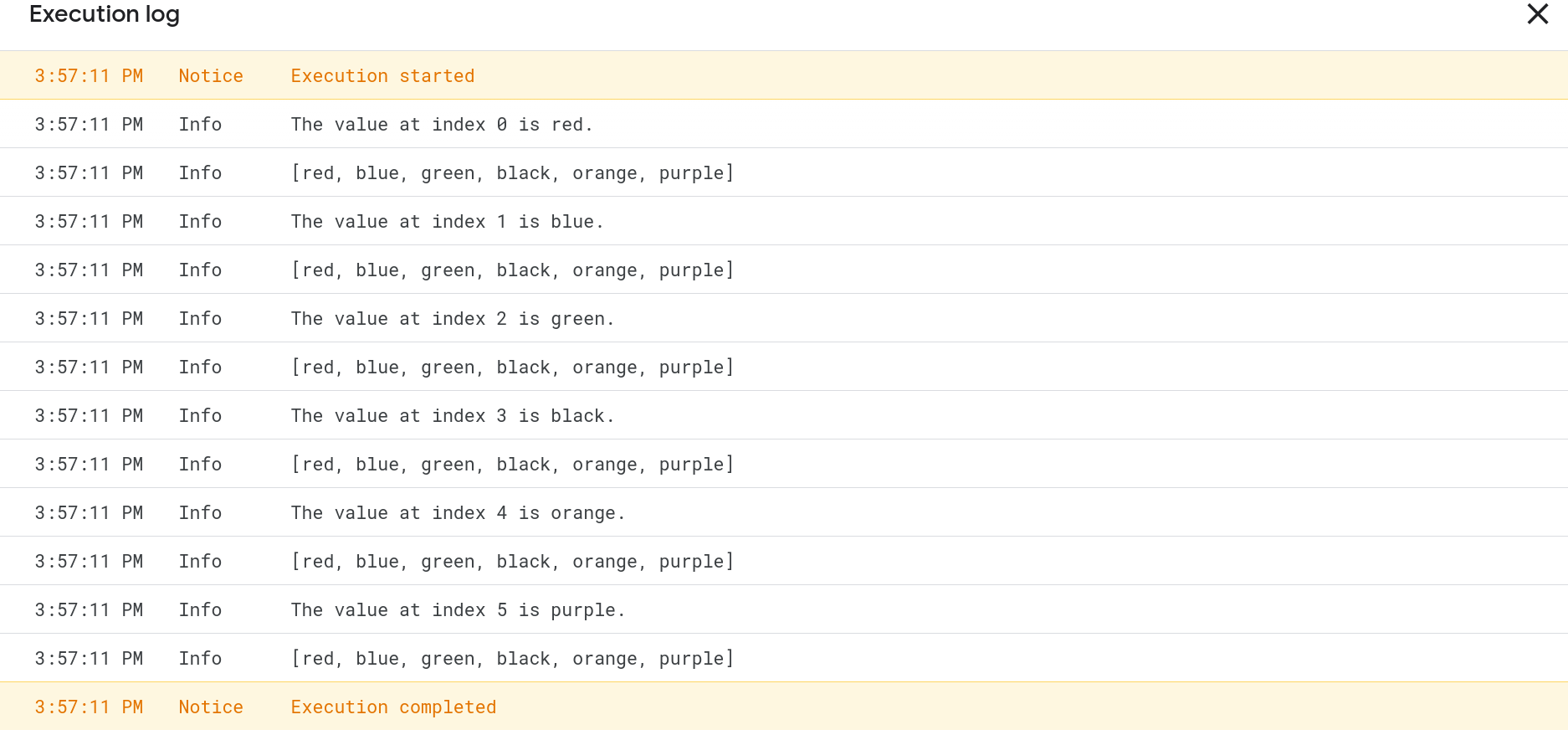
Example 4: The forEach method will skip undefined values in an array
If an array has an undefined value, the forEach()
method will skip it and the callback function will never be called.
In the code below, the array colors
has an undefined value between the elements "red"
and "green"
. When you use the forEach()
method, this element will be skipped and the callback function will not be called for this value.
Therefore, when you see the logged output, you will not see an undefined value. The code also increments a counter called count
every time the callback function is called. This counter will also not be incremented for this undefined value. Therefore, the value of the counter at the end of the forEach()
loop will be one less than the length of the array colors
. This discrepancy arises because the forEach()
method skips the undefined value in the array.
function forEachEx4() {
var colors = ["red",, "green", "black", "orange", "purple"];
var count = 0;
colors.forEach(function(value) {
Logger.log(value);
count++;
});
Logger.log(colors.length);
Logger.log(count);
}
Output
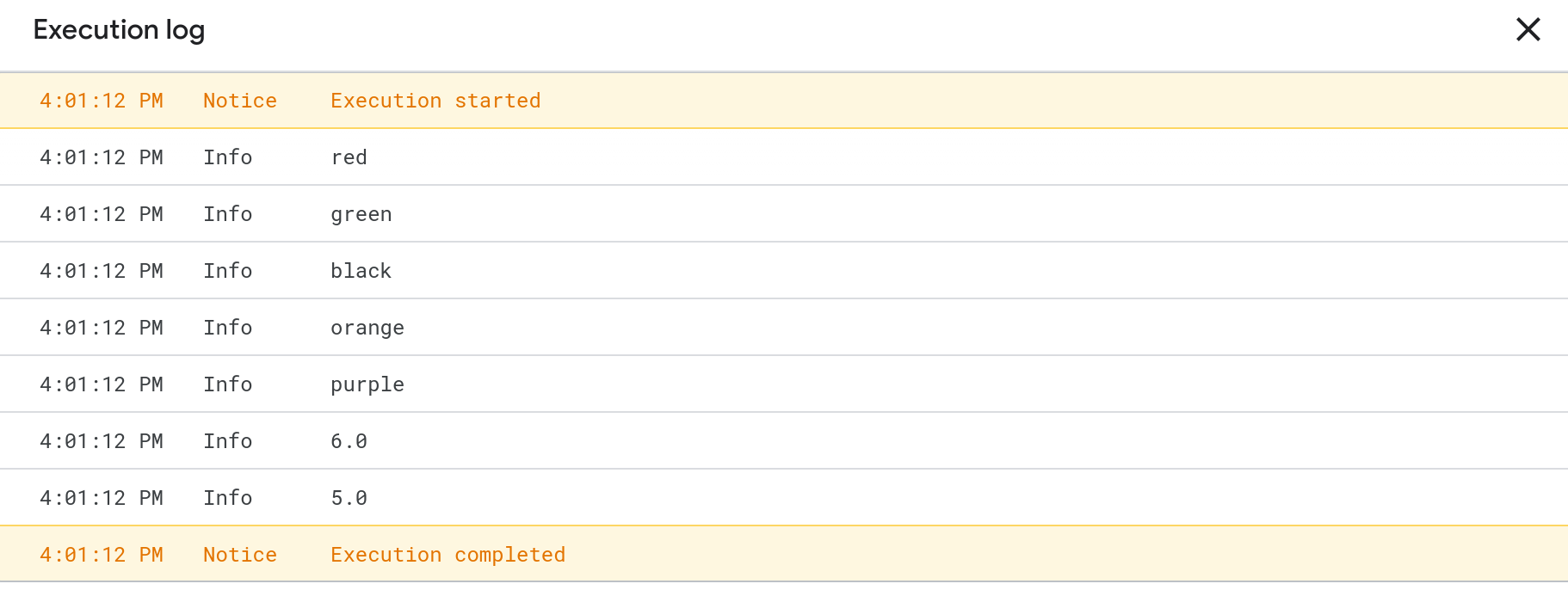
Example 5: The forEach method will always return undefinedfunction forEachEx5() {
var colors = ["red",, "green", "black", "orange", "purple"];
var count = 0;
var result = colors.forEach(function(value) {
Logger.log(value);
});
Logger.log(typeof result);
}
function forEachEx5() {
var colors = ["red",, "green", "black", "orange", "purple"];
var count = 0;
var result = colors.forEach(function(value) {
Logger.log(value);
});
Logger.log(typeof result);
}
Output
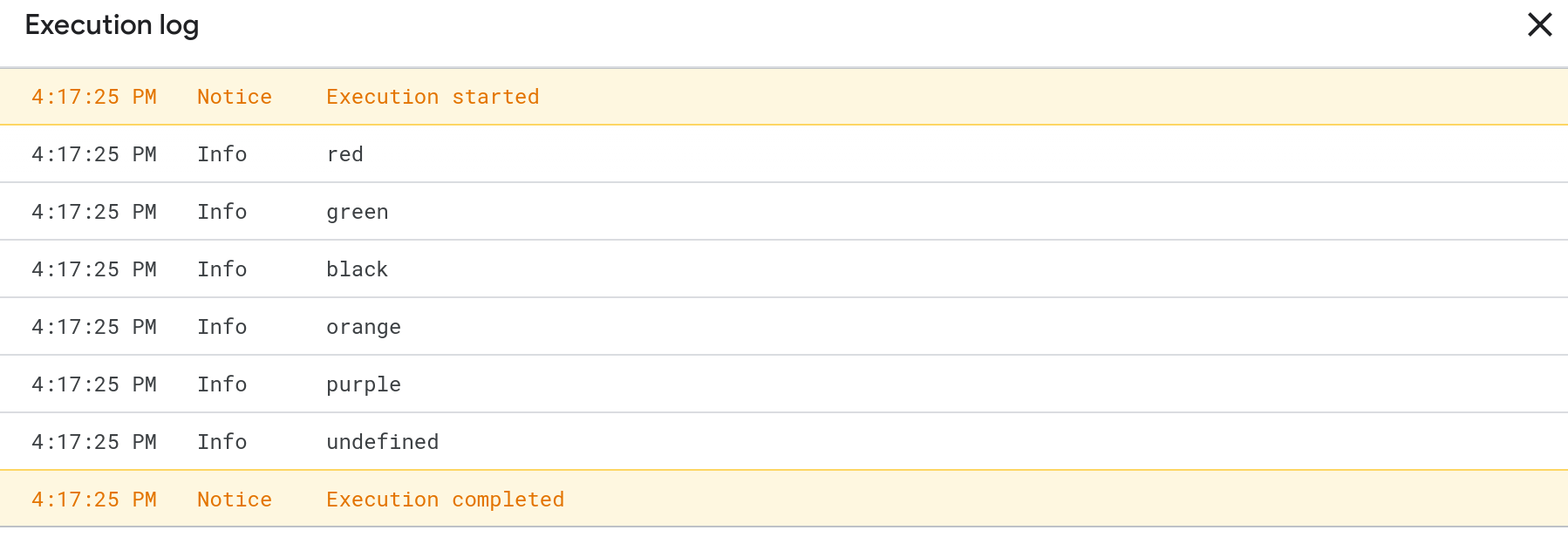
Example 6: You cannot break out of a forEach() loop
Unlike the for
loop or the while
loop, you cannot break out of a forEach
loop using the break
keyword. The forEach() method will always process every element in an array that is not undefined
. The only way to break out of the forEach
loop during execution is by throwing an error. This is not advisable. If your use case needs the ability to break out of a loop, please do not use the forEach
loop.
Not advisable: Breaking out of a forEach loop by throwing an exception
The code below breaks out of the forEach
loop when it encounters the value green by throwing an error. This could cause your entire program to fail unless the error is handled correctly. If you need the ability to break out of a forEach
loop, then you should use a different type of looping construct instead.
function forEachEx6() {
var colors = ["red","blue", "green", "black", "orange", "purple"];
colors.forEach(function(value) {
if(value === "green") {
throw "Encountered green so breaking out of the loop.";
}
Logger.log(value);
});
}
Output
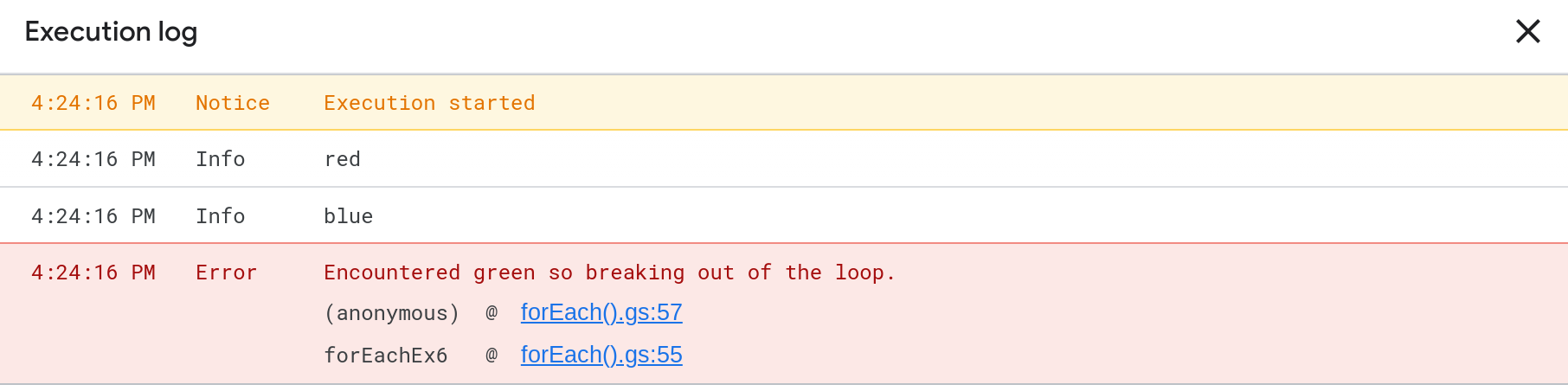
Conclusion
In this tutorial, you learned how to use the forEach()
method of an array to take some action on each of its elements. The forEach()
method is also known as the forEach()
loop because it is a convenient way to loop through every element in an array.
How was this tutorial?
Your feedback helps me create better content
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
By subscribing, you agree to our Privacy Policy and Terms of Service