Email notifications for Google Forms
In this tutorial, I will show you how to use Google Apps Script to automatically send an email notification whenever a Google Form is submitted. I will also show you how to include the contents of the form submission in the body of the email.
Google Forms already lets you set up form submission notifications but these only tell you that your form has received a new submission. They do not tell you what the contents of the submission were. So whenever you receive one of these notifications, you have to open the form (or the spreadsheet) to see what was submitted. This process can be cumbersome if you receive a lot of form submissions.
With a few lines of Google Apps script, you can write your own custom notification system that will also include the contents of the form submission in the email you receive.
For example, consider the form below with two questions.
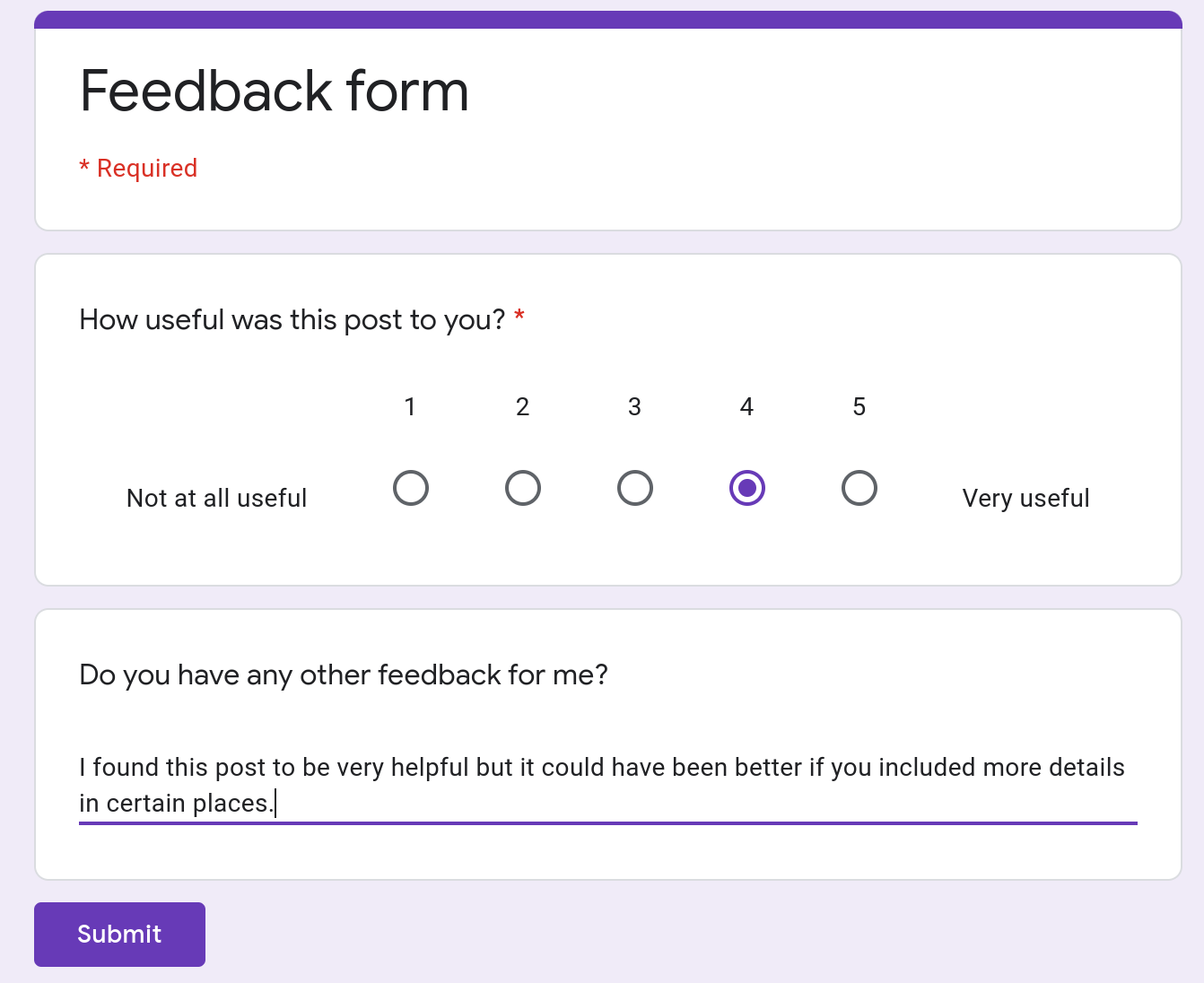
When you're done with this tutorial, submitting the above form will trigger an email notification like the one below that will tell you that the form was submitted and will include the user's responses in-line in the email itself. You don't have to open the form or its associated spreadsheet to see what was submitted. Isn't that nifty?
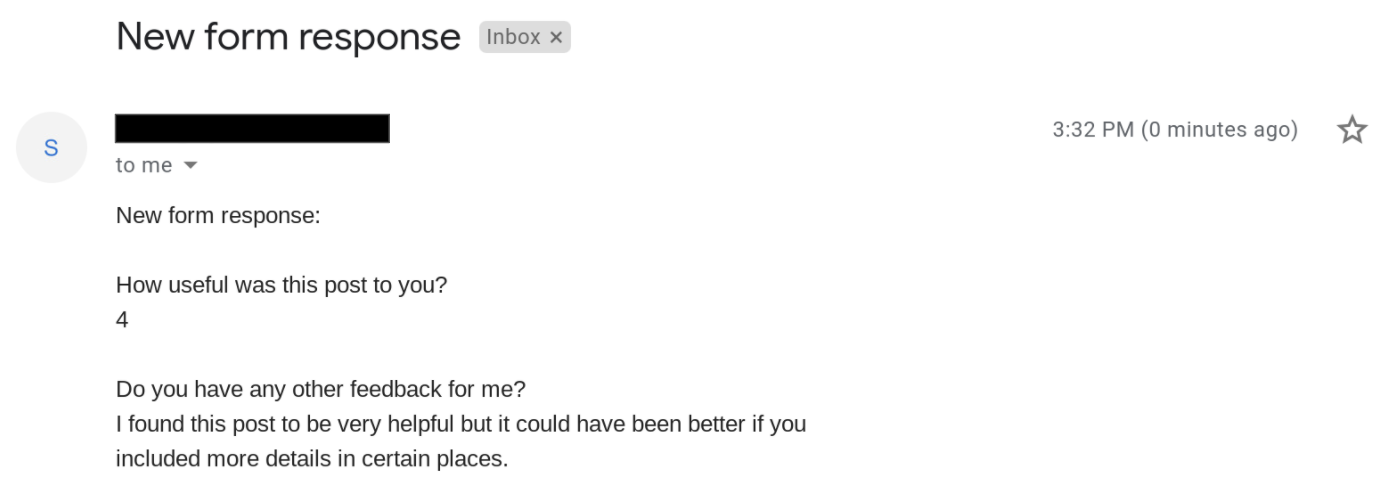
Prerequisites
This tutorial assumes that you are familiar with:
Creating simple Google Forms.
The concept of triggers and how to create them.
5 steps to automatically send email notifications whenever a Google Form is submittedStep 3 — Create an installable trigger to run a function whenever the form is submitted
Step 4 — Process the form submission and send out an email notification
Step 5 — Submit the form and see if you receive an email notification
Step 1 — Create the Google Form
Step 3 — Create an installable trigger to run a function whenever the form is submitted
Step 4 — Process the form submission and send out an email notification
Step 5 — Submit the form and see if you receive an email notification
The first step is to create the Google Form. For the purposes of this tutorial, I created a really simple one with just two questions. The first question asks users to rate how helpful a blog post was on a scale of 1 to 5. The second question allows readers to provide optional feedback on the blog post.
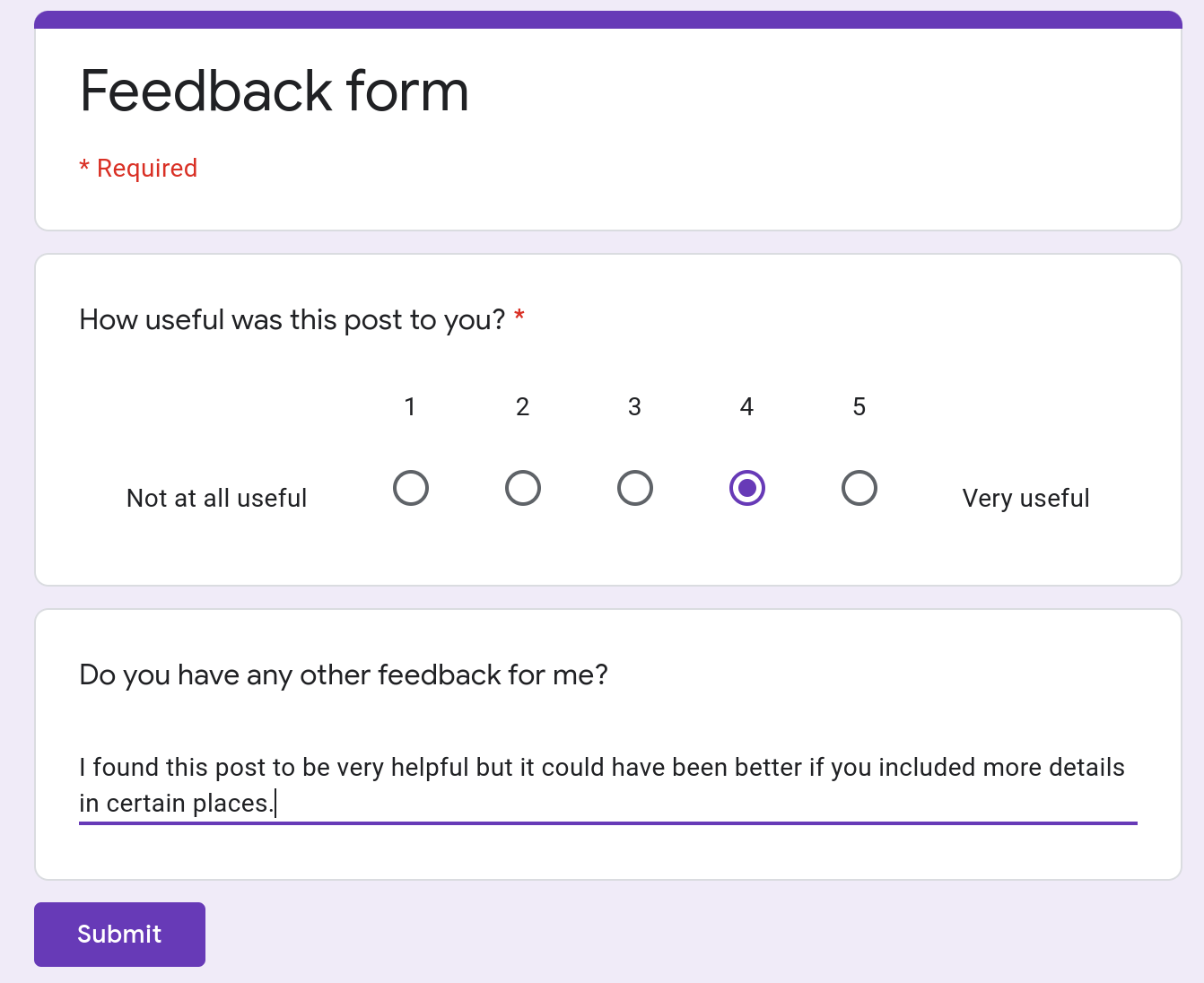
Step 2 — Open the Apps Script editor from the form
Next, open the Apps Script editor from the Google Form. Here is a video that shows you how to do that.
Step 3 — Create an installable trigger to run a function whenever the form is submitted
Our objective is to send an email notification whenever the form is submitted. Therefore, we need some way to automatically trigger (i.e., cause) this notification to be sent whenever the form is submitted. To achieve this, we will create an installable trigger that runs a function called onFormSubmit()
whenever a user submits the Google Form.
// Restrict the script's authorization
// to the form it is bound to.
//@OnlyCurrentDoc
// Create a form submit installable trigger
// using Apps Script.
function createFormSubmitTrigger() {
// Get the form object.
var form = FormApp.getActiveForm();
// Since we know this project should only have a single trigger
// we'll simply check if there are more than 0 triggers. If yes,
// we'll assume this function was already run so we won't create
// a trigger.
var currentTriggers = ScriptApp.getProjectTriggers();
if(currentTriggers.length > 0)
return;
// Create a trigger that will run the onFormSubmit function
// whenever the form is submitted.
ScriptApp.newTrigger("onFormSubmit").forForm(form).onFormSubmit().create();
}
Try running the above code. When you do, you'll be asked to authorize your script. Upon authorization, your trigger will be created. You can confirm this by navigating to the triggers page in the Apps Script editor. Here is a video that shows you how to do that.
If you run the createFormSubmitTrigger()
function again, nothing should happen since the trigger already exists and the function will return without taking any action. This is because currentTriggers.length
will now be 1, which is greater than 0.
Step 4 — Process the form submission and send out an email notification
When a user submits the form, the form submission trigger will fire and it will run the onFormSubmit()
function per the configuration we specified in the previous step.
Next we need to write the code for this function. This function will process the form submission and send out the email notification.
The first thing to know is that when the onFormSubmit()
function is called, Apps Script will pass it an "event" object containing the user's response. We will then use this information to create the email notification.
In addition to the onFormSubmit()
function, we will also create a sendEmail()
function that takes the body of the email as a parameter and sends the email to the email address you specify.
Note: Please remember to replace <EMAIL_ADDRESS>
in the sendEmail()
function with the email address where you want notifications to be sent.
// A function that is called by the form submit
// trigger. The parameter e contains information
// submitted by the user.
function onFormSubmit(e) {
// Get the response that was submitted.
var formResponse = e.response;
// Get the items (i.e., responses to various questions)
// that were submitted.
var itemResponses = formResponse.getItemResponses();
// Create a variable emailBody to store the body
// of the email notification to be sent.
var emailBody = "New form response:\n\n";
// Put together the email body by appending all the
// questions & responses to the variable emailBody.
itemResponses.forEach(function(itemResponse) {
var title = itemResponse.getItem().getTitle();
var response = itemResponse.getResponse();
emailBody += title + "\n" + response + "\n\n";
});
// Send the email notification using the
// sendEmail() function.
sendEmail(emailBody);
}
// A function that sends the email
// notification.
function sendEmail(emailBody) {
MailApp.sendEmail("<EMAIL_ADDRESS>", "New form response", emailBody);
}
The full script
When you are done with step 4, your script should have the below code in it. Please do feel free to tweak it based on your use case. I'm including the full script in one place to make it easy for you if you're following this tutorial step by step.
Note: Again, please remember to replace <EMAIL_ADDRESS>
in the sendEmail()
function with the email address where you want notifications to be sent.
// Restrict the script's authorization
// to the form it is bound to.
//@OnlyCurrentDoc
// Create a form submit installable trigger
// using Apps Script.
function createFormSubmitTrigger() {
// Get the form object.
var form = FormApp.getActiveForm();
// See if the trigger has already been set up.
// Since we know this project should only have a single trigger
// we'll simply check if there are more than 0 triggers. If yes,
// we'll assume this function was already run so we won't create
// a trigger.
var currentTriggers = ScriptApp.getProjectTriggers();
if(currentTriggers.length > 0)
return;
// Create a trigger that will run the onFormSubmit function
// whenever the form is submitted.
ScriptApp.newTrigger("onFormSubmit").forForm(form).onFormSubmit().create();
}
// A function that is called by the form submit
// trigger. The parameter e contains information
// submitted by the user.
function onFormSubmit(e) {
// Get the response that was submitted.
var formResponse = e.response;
// Get the items (i.e., responses to various questions)
// that were submitted.
var itemResponses = formResponse.getItemResponses();
// Create a variable emailBody to store the body
// of the email notification to be sent.
var emailBody = "New form response:\n\n";
// Put together the email body by appending all the
// questions & responses to the variable emailBody.
itemResponses.forEach(function(itemResponse) {
var title = itemResponse.getItem().getTitle();
var response = itemResponse.getResponse();
emailBody += title + "\n" + response + "\n\n";
});
// Send the email notification using the
// sendEmail() function.
sendEmail(emailBody);
}
// A function that sends the email
// notification.
function sendEmail(emailBody) {
MailApp.sendEmail("<EMAIL_ADDRESS>", "New form response", emailBody);
}
Step 5 — Submit the form and see if you receive an email notification
The final step is to test your script. Try submitting your form and see if you receive an email notification at the email address you specified in the sendEmail()
function. The notification should contain your responses to the questions on the Google Form.
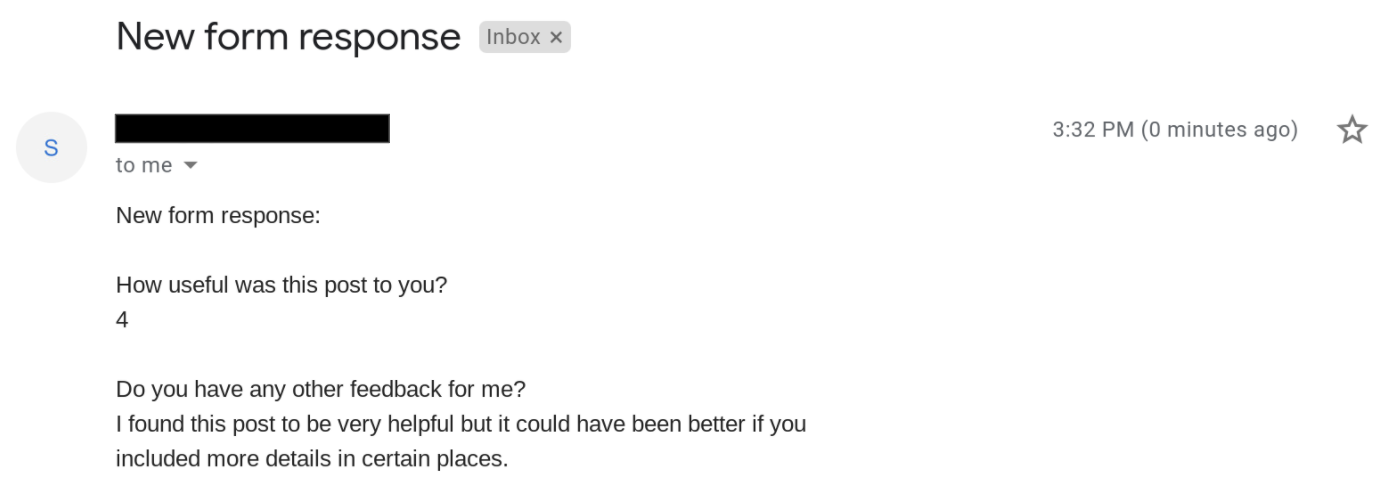
Conclusion
In this tutorial I showed you how to automatically send email notifications whenever a Google Form is submitted and also include the contents of the submission in the notification itself.
Hope you found this tutorial helpful. Thanks for reading!
DISCLAIMER: This content is provided for educational purposes only. All code, templates, and information should be thoroughly reviewed and tested before use. Use at your own risk. Full Terms of Service apply.
Small Scripts, Big Impact
Join 1,500+ professionals who are supercharging their productivity with Google Sheets automation
By subscribing, you agree to our Privacy Policy and Terms of Service